Split python: the most powerful method for string manipulation

Split in Python is a versatile tool that allows you to split a string into multiple substrings. Based on a specified separator. Therefore, this function is essential in a wide range of applications, such as word processing, data analysis and web development. When we need to extract specific words or phrases from a text. Separate data elements from a CSV file, or divide a web page into its component parts. The split function is an indispensable when learning to work in Python.
In this article, we will explore in depth the concept of split Python and how it works. Let’s learn how to implement split
Python in our code and see examples. Of how the function can be applied to work with strings
. Furthermore, we will discuss the benefits and limitations of this technique and explore alternatives to split Python.
Table of Contents
What is split in Python?
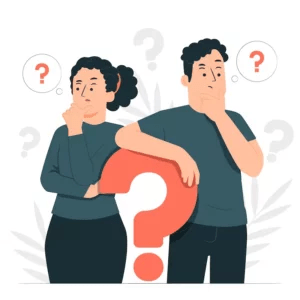
The “split” function is an operation in Python that allows you to split a string into a list of substrings, based on a specific pattern. The “split
” function takes as an argument the original string and a pattern that indicates how the string should be divided. The pattern can be a string or a list of strings, and can contain multiple entries separated by commas to indicate that the input string should be split
into a list of substrings based on each of these entries. The “split
” function can be used to split strings based on whitespace, tabs, specific characters, or even regular expressions.
Syntax
The split
() method is used to divide a string into a set of substrings , based on a certain criterion. Thus, the syntax of the split() method is as follows:
split(sep[, maxsplit])
- The argument
sep
is the criteria used to divide the string. Thus, it can take the value of a string, a set of strings, a regular expression, or a regular expression with a backreference function. - The argument
maxsplit
is optional and indicates the maximum number of substrings that will be returned. If not specified, the function will continue splitting the string until there are no more delimiters.
The sep criterion can be a simple string, such as ” “, which divides the string into words separated by whitespace. This way, it can also be a set of strings, like ” ‘. ,;:”, which can separate the string into words that contain these specific characters entered in the code.
Furthermore, it is possible to use regular expressions as division criteria. For example, the following line of code splits the string into words that contain uppercase letters:
words = text.split(r'[A-Z]')
Therefore, the max split
argument is optional and indicates the maximum number of substrings that are returned. If not specified, the function will continue splitting the string until there are no more delimiters. For example, the following line of code splits the string into just two words:
phrases = text.split("'", maxsplit=1)
Another example, where the max split
argument is being used to divide the string into three words:
phrases = text.split("'", maxsplit=3)
It is important to remember that the split
() method returns a list of strings, therefore, we need to check that the string is divided correctly.
Benefits of split python
Split in Python offers several benefits to splitting strings. Here are some of the main benefits:
- Flexibility: The “split” function allows you to split strings based on several different patterns. Such as whitespace, tabs, specific characters or regular expressions. This allows you to split your strings according to your project’s specific needs.
- Efficiency: The “split” function is a fast and efficient operation in Python. Especially when compared to other ways of splitting strings. Such as using loops or search functions.
- Simplicity: The “split” function is a simple and easy-to-use operation in Python. Just pass the original string and the split pattern as arguments to the function. And it returns a list of substrings.
- Reusability: The “
split
” function can be easily reused in different parts of your code. Which helps maintain consistency and organize the code. - Data Manipulation: The “
split
” function can be used to split strings into substrings. Which can be useful for manipulating data in a string. Such as removing or adding words in a sentence. Or splitting a string into separate fields in a table of data.
How to implement Python split in your code
Split is a function in Python that allows you to divide a string or list into two or more parts. To use split in Python. You can call the function with an argument. That specifies the separator you want to use to split the string or list .
For example, if you want to split a string “hello world” into two parts, you can use split as follows. As in this example, the separator used is a white space (““ ). Then the split function will return a list of the two words in the original string. look:
text = "hello world"
words = text.split(" ")
To split
a list into two or more parts, you can use the same technique:
text = ["hello", "world"]
words = list.split(",")
In this case, the separator used is a comma (“,”). So the split
function will return a list with the two words from the original list.
It is important to remember that split
may return an empty list if the string or list has no elements. For example, if you use split
with an empty string (“”), the function will return an empty list.
Additionally, you can specify the number of parts you want to divide the string or list into. For example:
text = "hello world"
words = texto.split(maxsplit=2)
In this case, the split
function will return a list of the two words in the original string. And the max split
=2 argument specifies that the function should stop splitting
the string after two elements.
Implementing split in Python
Split in Python
can be implemented in several ways. But one of the most common ways is using the “split” method of the str (string) object. Which is one of the basic classes in Python.
The “split” method takes a delimiter (or separator) as an argument and returns a list of all substrings (or fragments). Of the original that were separated
by the delimiter. The delimiter can take the form of a string or a regular expression.
For example, to split a string into a set of substrings using a whitespace delimiter. We can use the following code:
text = "Python is an open source programming language"
words = text.split()
print(words)
The output would be:
['Python', 'is', 'a', 'language', 'of', 'programming', 'of', 'source', 'open']
Another example, to split a string into a set of substrings using a comma delimiter. We can use the following code:
text = "Python is an open source programming language"
words = text.split(',')
print(words)
The output would be:
['Python', 'is', 'a', 'language', 'of', 'programming', 'of', 'source', 'open']
You can also use regular expressions to implement split. For example, to split a string into a set of substrings based on a specific character pattern. We can use the following code:
text = "Python is an open source programming language"
words = re.split(r'[^\w\s]', text)
print(words)
The output would be:
['Python', 'is', 'a', 'language', 'of', 'programming', 'of', 'source', 'open']
The code above uses Python’s “re” (regular expressions). Library to split the string based on a character pattern that excludes all characters that are not letters. Numbers, or whitespace.
It is important to note that split
can be used in many other situations. From splitting a string into a set of words to extracting specific information. From a string using delimiters or regular expressions.
Advanced usage examples
Split
in Python
can be used in many situations
. For example to split a string into a set of substrings based on a specific delimiter. Let’s consider some examples that use the “split” method in conjunction with other Python control structures. Such as input, switch case , while, for , len and range .
- Input:
We use the input method to read a user input. Let’s consider the following example:
text = input("type a sentence: ")
sentence = text.split()
print(sentence)
Thus, when executing
the code above, the user will be asked to type a sentence. The phrase entered by the user is being split into a set of words using the “split
” method and the output will be:
['type a sentence:']
- Switch case:
We can execute different blocks of code based on a specific value with this Python control framework that allows . So let’s consider the following example:
text = "Python is an open source programming language"
phrases = text.split()
for phrase in phrases:
if phrase == "Python":
print("It's a language")
elif phrase == "It is":
print("It is")
elif phrase == "a":
print("a")
elif phrase == "language":
print("language")
elif phrase == "the":
print("the")
elif phrase == "programming":
print("programming")
elif phrase == "code":
print("code")
elif phrase == "open":
print("open")
Running the code, the output will be:
It's a language
- While:
The while is a control structure that allows executing a block of code as long as a specific condition is true. Let’s consider the following example:
text = "Python is an open source programming language"
phrases = text.split()
counter = 0
while counter < len(phrases):
print(phrases[counter])
counter += 1
Running the code, the output will be:
python
It is
one
language
in
schedule
in
code
open
Limitations of split Python
The “split” method in Python. Is a powerful tool for splitting a string into a set of substrings based on a specific delimiter. However, there are some limitations to be considered. Some of these limitations include:
- Duplicate delimiters:
If the delimiter specified for the method appears twice in the original string. The first occurrence will be used as the delimiter and the rest of thestring
is being ignored. - Delimiters that are not unique:
If the delimiter specified for the method is not unique (that is, it can also appear in the original string as part of a substring). The original string will not besplit
correctly. - Limitation on strings that contain escape characters:
If the originalstring
contains escape characters, such as “\n” or “\t”, the interpretation of the delimiter will be affected. - Limitation on strings containing Unicode characters:
If the original string contains Unicode characters, the method may not work correctly. - Limitation on strings containing empty substrings:
If the original string contains empty substrings, the method can ignore them or include them as part of other substrings.
Thus, it is important to consider these limitations when using the method. And always verify that the original string meets the necessary requirements for a correct division. In some cases, it may be necessary to use other string processing tools. Such as the “replace” method or the “re” library. To deal with these limitations.
Alternatives to Split Python
Unlike split
in other languages, split in Python is implemented directly in Python. But there are some alternatives that can be considered in certain situations
.
- String manipulation using regular expressions: Instead of using the split method. You can use regular expressions to extract substrings from a string. This is especially useful when you need to extract a series of substrings based on a specific regular expression. For example:
import re
text = "Hello World! There are many examples of strings in Python, but split is one of the best ways to split a string into substrings."
# Extract all whitespace using regular expressions
phrases = re.findall(r'\S+', text)
print(phrases)
- Using external libraries: There are some libraries that provide string processing capabilities that can be used as alternatives to
split
. For example, Python’s “re” library provides functions to manipulate strings using regular expressions. And the Pandas anda numpy library can be used tostrings
into substrings based on a given pattern. Splitting
strings into lists using other methods: Although the method is the most commonly used method forsplitting
strings into lists. Other functions can be used tosplit
strings into substrings. For example, the “splitlines” method can be used tosplit
a string into lists of lines. And the “partition” method can be used tosplit
a string into three parts based on a specific character.
However, it is important to remember that these alternatives may have lower performance compared to the split method. Which may be directly implemented in Python
.
Conclusion
In short, split()
is a useful function in Python to split
a string into smaller substrings based on a specified character separator. The function is easy to use and offers several options for handling guarantees and ignoring special characters.
The split()
is an efficient and fast function, making it suitable for handling large amounts of data. Although it is not the only option available in Python for splitting
strings. The function is flexible enough to be used in a variety of contexts, from data analysis to text manipulation.