While in Python: The Complete Guide to Repetitive Execution
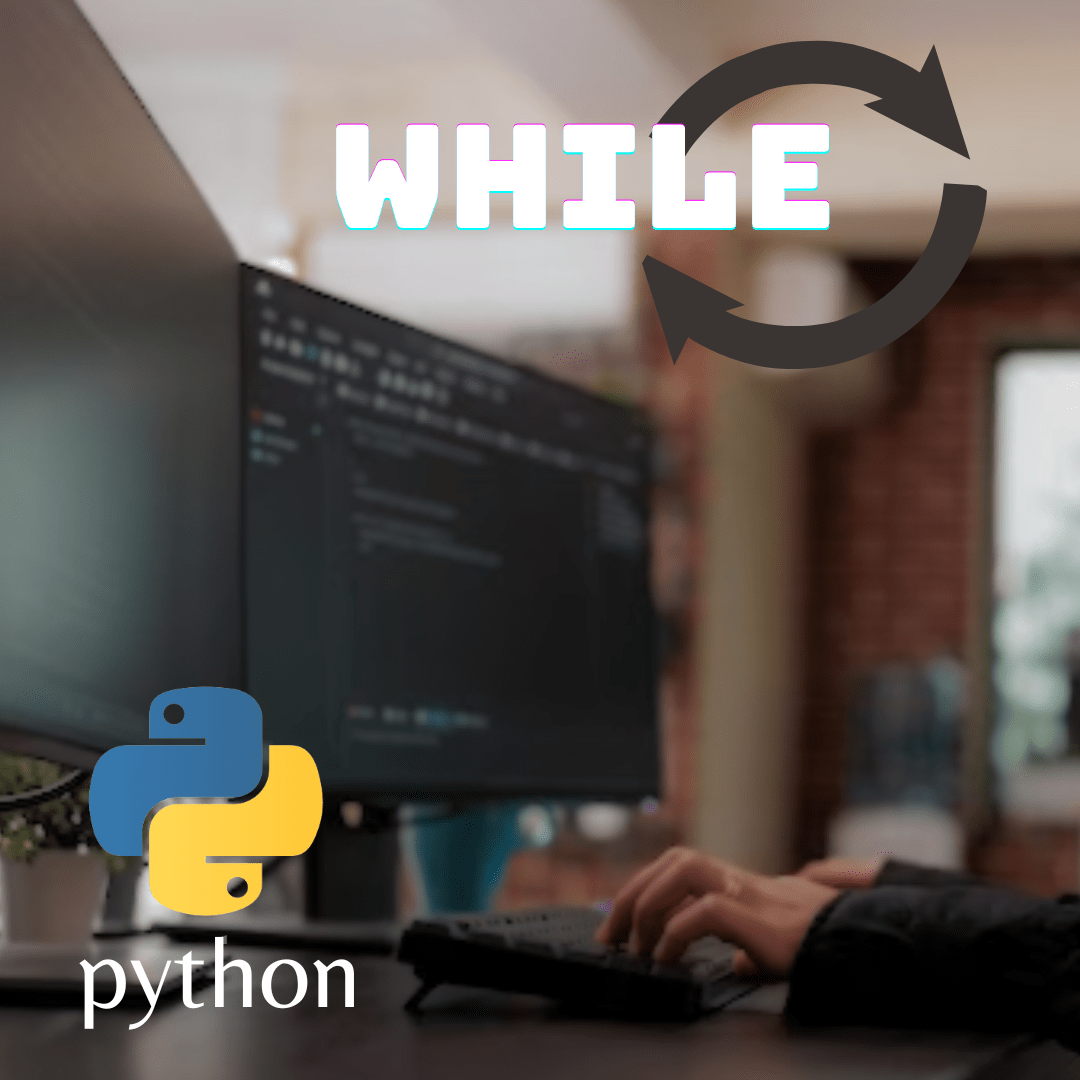
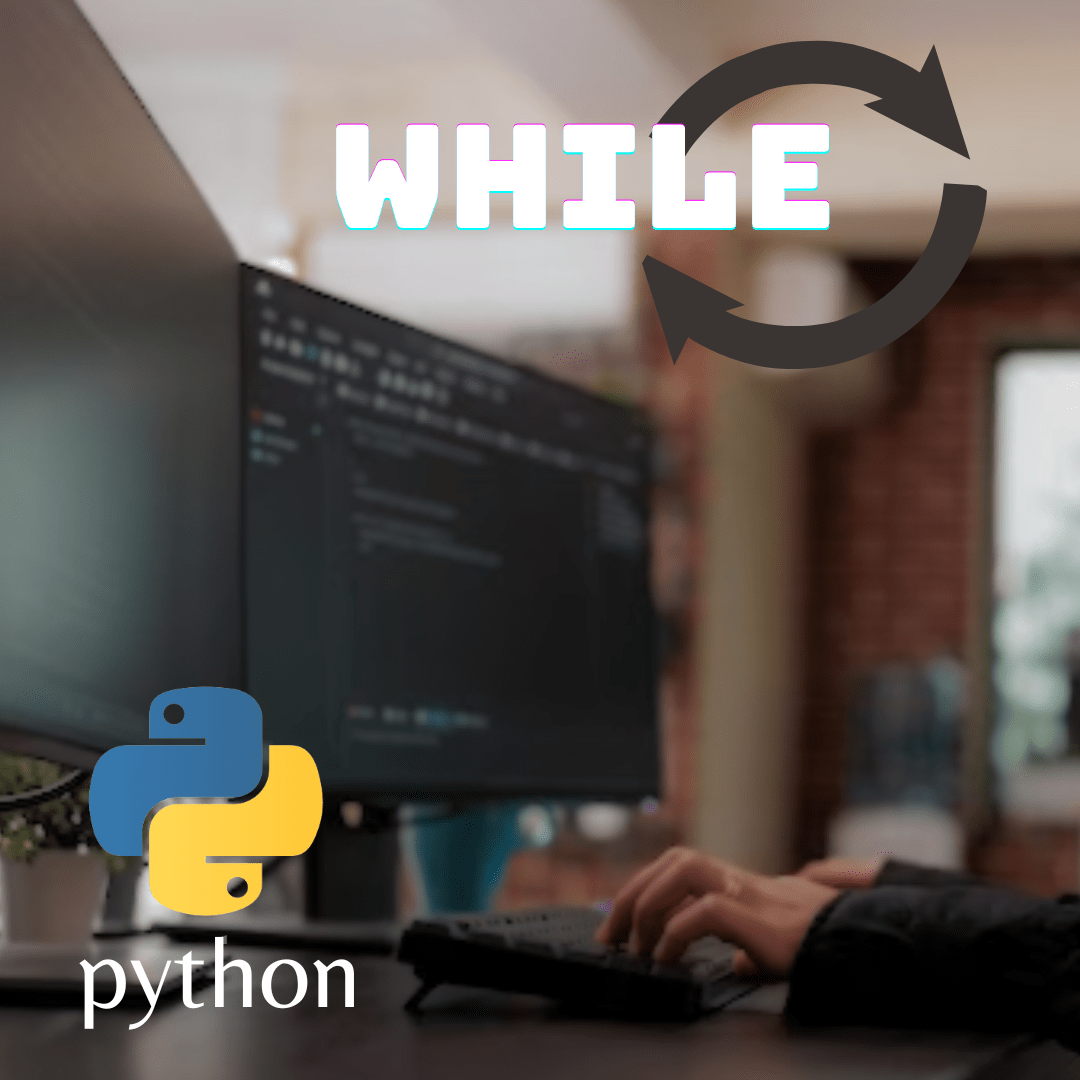
The while loop is a flow control structure in Python that lets you execute a block of code repeatedly until a certain condition is true. Still, this type of loop is useful when you know exactly how many times you want to run a block of code before it stops.
We can define a function using just a sentinel and a body of code. However, we check the sentinel condition before each code block execution, and if it is true. We execute in the code. The loop continues executing the code until the condition becomes false. Furthermore, this flow control framework is very powerful and we find its use in many types of programs, from simple scripts to complex applications. The while loop is especially useful in situations where you need to repeat the execution of a block of code until it reaches a certain state.
In this article, we’ll explore the use of the while loop in Python, including its syntax, conditions, and flow control. In addition, we will examine practical examples of using the while loop in Python and compare it with other types of loops available in Python. So let’s start!
Table of Contents
while loop syntax in Python
The while syntax in Python is quite simple. Likewise, we run a sentinel before each iteration of the loop and run code if the sentinel is true. The loop continues executing the body of code until the sentinel becomes false.
The basic syntax of the while loop in Python is as follows:
while condition:
# code block to be executed
The condition checks a boolean expression before each iteration of the loop. If the condition is true, we execute the block of code between the quotation marks. The loop continues executing the code inside the block until the condition becomes false.
You can add a counter to track the number of loop iterations. To do this, we use a variable and check its value inside the condition. In this example, the loop will execute the code block inside the quotes whenever counter is less than 10. The counter will be incremented in each iteration of the loop, See:
counter = 0
while counter < 10:
# code block to be executed
counter += 1
In short, the while loop syntax in Python is quite simple and powerful, allowing you to run a block of code repeatedly until a certain condition is true.
Simple examples of using the while loop in Python
The while loop is one of the simplest and most used loops in Python. This way, the code runs repeatedly as long as a given condition is true. To use the while loop, you need to define a condition that will evaluate before each iteration.
Here are some simple examples of how to use the while loop in Python:
Countdown:
In this example, the condition i < 5
is evaluated before each iteration, and the value of i
is incremented by 1 with each iteration.
i = 0
while i < 5:
print(i)
i += 1
The result is a countdown from 0 to 4.
Checking a keyword:
In this example, the condition is evaluated before each iteration, and the input prompt is redisplayed if the string word not in ["key1", "key2"]
type keyword entered is invalid. The loop will run continuously until you enter a valid keyword.
word = input("Insert a keyword: ")
while word not in ["key1", "key2"]:
print("Please enter a valid keyword!")
word = input("Insert a keyword: ")
Simple calculator:
In the example, the condition True
is used to create an infinite loop. As the user enters an operation, the value of soma
, subtração
, multiplicação
or divisão
is updated and the corresponding output is displayed. If the user enters an invalid operation, the output “Invalid operation!” is displayed and the loop is interrupted with the instruction break
.
while True:
s1 = float(input("Insert a number: "))
s2 = float(input("Insert other number: "))
sum = s1 + s2
subtraction = s1 - s2
multiplication = s1 * s2
division = s1 / s2
print("Sum:", sum)
print("Subtraction :", subtraction)
print("Multiplication:", multiplication)
print("Division:", division)
operation = input("Insert a operation: +, -, * or / ")
if operation == "+":
s1 = float(input("Insert the first number: "))
s2 = float(input("Insert the second number: "))
sum = s1 + s2
print("Sum:", sum)
elif operation == "-":
s1 = float(input("Insert the first number: "))
s2 = float(input("Insert the second number: "))
subtraction = s1 - s2
print("Subtraction:", subtraction)
elif operation == "*":
s1 = float(input("Insert the first number: "))
s2 = float(input("Insert the secund number: "))
multiplication = s1 * s2
print("Multiplication:", multiplication)
elif operation == "/":
s1 = float(input("Insert the first number: "))
s2 = float(input("Insert the second number: "))
division = s1 / s2
print("Division:", division)
else:
print("Operation invalid!")
break
Sum length:
In this example, we have the while loop along with the function len
where the condition len(soma) < 5
is evaluated before each iteration. The variable soma
is initialized to 0, and the iteration will continue to execute until the length of soma
is equal to 5. As the loop executes, the variable soma
is incremented by 1 on each iteration, see:
sum = 0
while len(sum) < 5:
sum += 1
print(sum)
The result is a loop that increments the value of soma
until the length of soma
is equal to 5.
While Loop Conditions in Python
There are several ways to define a condition for the while loop. Here are some examples:
boolean condition
It’s the most common way to define a condition for the while loop in Python. The while loop involves a Boolean expression that evaluates to true or false on each iteration of the loop. If the expression is true, the loop continues; if false, the loop ends.
For example, here is the Boolean condition x < 10
is evaluated on each iteration of the while loop. Since x
it is less than 10, the expression is true and the loop continues.
x = 0
while x < 10:
print(x)
x += 1
comparison operators
In addition to boolean expressions, we can also use comparison operators to define a condition for the while loop. For example, the condition of the while loop is x < 10 or x > 0
. The expression x < 10
is true, so the condition is true, and the loop continues. When it x
reaches 10, the expression x > 0
is true and the condition is again true, see below:
x = 0
while x < 10 or x > 0:
print(x)
x += 1
border check
In addition to comparing to a specific number, you can use bounds checking to define a condition for the while loop. For example, the condition of the while loop is x < 10 or x >= -5
. The expression x < 10
is true, so the condition is true, and the loop continues:
x = 0
while x < 10 or x >= -5:
print(x)
x += 1
while loop flow control in Python
The while loop flow control in Python is a flow control structure that executes a sequence of statements repeatedly until a condition is true. While the while loop is simple in concept. We can use it in complex ways to manage loop execution and ensure that the condition is true only once.
A common strategy to ensure that the condition is true only once is to use a control variable to track the number of loop iterations. For example, a control variable i
is used to track the number of iterations of the while loop. When it i
reaches 5, the loop condition is stopped using the keyword break
:
i = 0
while i < 10:
if i == 5:
break
i += 1
This way, the condition is true only once, when i
it is equal to 5.
More advanced While examples in python
The while loop is a versatile solution to many problems in different contexts. In that vein, here are some examples of how we use the loop to solve specific problems like processing lists and matrices.
Example 1: Processing a list.
In this example, we use the while loop to increment all values in a list. We use the variable count
to track the number of elements in lst
and it is updated on each iteration of the while loop. So when it count
reaches the length of lst
, the loop condition is true and the loop terminates.
lst = [1, 2, 3, 4, 5]
count = 0
while count < len(lst):
lst[count] += 1
count += 1
print(lst)
The output will be:
[2, 3, 4, 5, 6]
Example 2: Process an array of numbers.
Using a while loop, we can process an array of numbers by multiplying each element by its position in the array and adding 1 to the column. Therefore, we process the matrix in reverse. Multiplying each element by its position in the matrix and adding 1 to the column. Look:
# Create a 3x3 matrix of numbers
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Loop while
while True:
# Loop through columns
for column in matrix:
# Multiply each element by the column
multiplying = 1
for element in column:
multiplying *= element
# Add 1 to column
for additional_column in matrix:
additional_column.append(1)
# Print the current matrix
print(matrix)
The result of running this code is:
[[1, 2, 3], [14, 15, 16], [21, 22, 23]]
[[2, 4, 6], [15, 18, 21], [22, 27, 32]]
[[3, 6, 9], [18, 24, 27], [30, 36, 42]]
Example 3: Finding the number that appears the fewest times
It is possible to find the number that appears the fewest times in a list. As shown below for an example:
# Create a list of numbers
list = [1, 2, 3, 2, 1, 4, 3, 2]
# Initialize counter to 0
counter = 0
# Initialize the smallest number to None
minor_number = None
# Loop while
while counter < len(list):
# Check if the number has already been counted
if counter not in counter_list:
# Update minor number
minor_number = counter
# increment the counter
counter += 1
# Prints the result
print("The number that appears the fewest times is:", minor_number)
Comparison with other loops in Python:
The while loop is one of the main loop types in Python, which allows you to execute a block of code repeatedly as long as a specific condition is true. However, Python also offers other types of loops, such as the for loop in python and the do-while loop. Which have some similarities and differences in terms of their syntax and usage.
However, below, we’ll look at the similarities and differences between the while loop, the for loop, and the do-while loop in Python:
Similarities:
- The three types of loops – while, for, and do-while – allow for the repeated execution of a block of code until we reach a specific condition.
- It is possible that we use the three types of loop – while, for and do-while – in conjunction with other flow control structures, such as if/else and switch/case.
- All three types of loops can process sequences of data such as lists, tuples, and strings.
Differences:
- The while loop executes while a specific condition is true. The for loop executes while the condition is true, and the do-while loop executes while the condition is true or the function’s output value is other than None.
- The while loop executes in the simplest and most straightforward way. Whereas the for loop requires you to explicitly specify control variables such as start and end. The do-while loop has an intermediate syntax between the while loop and the for loop.
- The do-while loop is best suited for situations where the condition is true on the first iteration of the loop while. Is best for situations where the condition does not become true on the first iteration of the loop.
The following are examples to illustrate the difference between the three loop types:
While loop example:
We use the while loop to print the values of x from 0 to 4. The loop condition, , x < 5
is true the first time the loop executes, which causes the code block inside the loop to execute. After the block of code executes, the variable x
is incremented by 1 and the loop condition is checked again. This occurs repeatedly until the condition x < 5
becomes false, that is, when x
it reaches the value 5, See:
x = 0
while x < 5:
print(x)
x += 1
For loop example:
We use the for loop in Python to print the values of range(5)
, which creates a sequence of numbers from 0 to 4. In this way, the structure for
is using the method range
em Python
to generate a sequence of numbers, and we use the variable i
to represent each number in the sequence. Therefore, the condition i < 5
is true for each value of i
in the sequence, which causes the block of code inside the loop to be executed once for each value of i
, See:
for i in range(5):
print(i)
Example do-while loop:
We use the do-while loop to print the values of x
0 through 4. The loop condition, x < 5
, is true the first time the loop executes, which causes the code block inside the loop to execute. Right after the block of code executes, the variable x
is incremented by 1 and the loop condition is checked again. Thus, this occurs repeatedly until the condition x < 5
becomes false, that is, when x
it reaches the value 5.
x = 0
do:
print(x)
x += 1
while x < 5
Conclusion
In summary, the while loop is a very powerful flow control structure in Python that allows you to execute a block of code repeatedly until a certain condition is true. It’s easy using the while loop, as we can define it with just a sentinel and a body of code.
Thus, the while loop becomes a better choice when we have prior knowledge of how many times we want to execute a block of code before stopping. It is especially useful in situations where we need to repeat code execution until we reach a certain state.
In this article, we discuss using the while loop in Python, including its syntax, conditions, and flow control, and provide practical examples of using the while loop in Python. When comparing with other types of loops available in Python. We realized that the while loop is a versatile and powerful flow control structure that we find in many types of programs.
We hope this article helped you better understand the use of the while loop in Python and how you can apply it in your own program creations. Also, learn about Switch Case and Scan in Python !