Len Python: complete guide to Python’s len() function
For sure! The len python function is undoubtedly one of the most used functions in the Python language, additionally it is used to return the length of an object , i.e. the number of elements contained in an object.
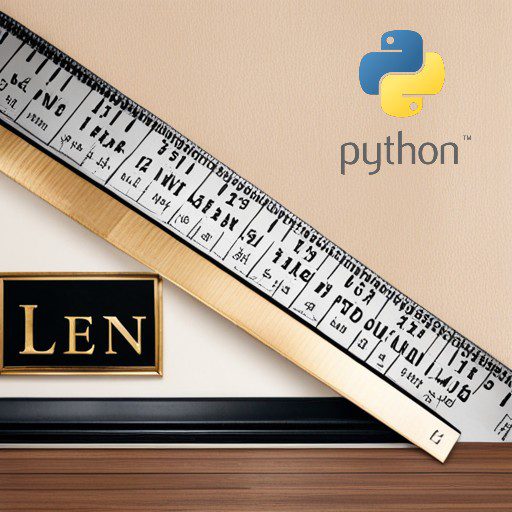
This functionality is fundamental for several applications, such as validating user input, calculating statistics, checking equality between iterable objects and even checking if at least one element of a list is true.
In this article, we are going to talk about the `len()` function, its parameters as object and base and how to use them correctly, code examples using the function how to calculate the size of a string, list, tuple, dictionary, set, file, and others, similar functions, fun facts about the function and where it is often used.
Table of Contents
How the len() function works
The `len()` function is a built-in Python function that returns the length of an object. The length of an object is the number of items it contains. The `len()` function can be used with many different data types, such as lists, tuples, dictionaries, strings, sets and more.
Syntax of len() function in python
The `len()` function syntax is simple. It only takes one argument, which can be a string or a collection. A sequence would be, for example, a string, list, tuple, dictionary, set or file. A collection would be a dictionary, set or frozenset. The function syntax is as follows:
len(objeto)
Where `object` is the object whose length you want to calculate.
What are len function parameters and how to use them correctly?
The len function in Python has only one mandatory parameter, which is the object. This parameter is the object whose size will be returned by the function. The object can be a string, list, tuple, dictionary, set, or any other iterable object in Python, as mentioned.
Furthermore, it is important to mention that the function len
has an optional parameter called base, which is only used when the object is a string that represents a number in a certain base, such as binary, octal or hexadecimal. It is worth mentioning that this parameter is added to the function’s mandatory parameter len
.
Example:
binary_number= "101010"
size = len(binary_number)
print(size)
# Output: 6
size = len(binary_number, 2)
print(size)
# Output: 6
In this example, you can specify the base parameter as 2 to indicate that the string “binary_number” represents a binary number. If you do not specify the base parameter, the “len” function will automatically assume that the string represents a number in base 10. It is important to remember that the base parameter is only relevant if the string represents a number in a base other than 10. If the input is not a string or if it is a string that represents a number in base 10, then the base parameter will have no effect.
In general, to correctly use the parameters of the len function in the python language, just pass the object whose size you want to get. If the object is a string representing a number in a base other than 10, you can specify the base parameter to get the correct size.
Examples of using the len() function
Let’s see some examples of how to use the `len()` function in Python:
Example 1 – Calculating the length of strings
In this example, we use the len() function to calculate the length of the text string. The length of the string is 2 characters including spaces, and the len() function returns the value 12.
text = "Hello, World!"
print(len(text))
# Output: 12
Example 2 – Calculating the length of lists
In this example, we use the function `len()` is to calculate the length of the list `list`. The length of the list is 5, which is the number of items it contains.
list = [1, 2, 3, 4, 5]
print(len(list))
# Output: 5
Example 3 – Finding the size of dictionaries
In this example, we use the `len()` function to determine the size of the dictionary, ie the number of key-value pairs there are. The length of the dictionary is 3, indicating that the dictionary has three elements.
dictionary = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
print(len(dictionary))
# Output: 3
Functions similar to len() in the python language
There are functions similar to len in Python, they are very useful for working with different data types and simplify coding. Among several similar functions here are some explanations of 10 functions and examples of applications:
Python’s count() function
The count() function Returns the number of occurrences of a given element in an iterable object. It is useful to be able to count how many times an element appears in a list, for example:
numbers = [1, 2, 3, 4, 2, 5, 2]
occurrences = numbers.count(2)
print(occurrences)
# Output: 3
In this example, we use the count é function to count the number of occurrences of the number 2 in the numbers list. The function returns 3 because the number 2 appears three times in the list.
function sum()
The sum function Returns the sum of the elements of an iterable object. It’s useful for summing the elements of a python list of numbers, for example:
numbers = [1, 2, 3, 4, 5]
sum = sum(numbers)
print(sum)
# Output: 15
In this example, we use the function sum is to add the elements of the list numbers. The function returns 15, which is the sum of the numbers 1 to 5.
Python max function
The max() function returns the largest element of an iterable object. It is useful for finding the largest number in a list, for example:
numbers = [1, 2, 3, 4, 5]
bigger = max(numbers)
print(bigger)
# Output: 5
In this example, we use the max function to find the largest number in the list of numbers. The function returns 5, which is the largest number in the list.
min() function
The min function Returns the smallest element of an iterable object. It is useful for finding the smallest number in a list, for example:
numbers = [1, 2, 3, 4, 5]
smaller = min(numbers)
print(smaller)
# Output: 1
In this example, we use the min is function to find the smallest number in the numbers list. The function returns 1, which is the smallest number in the list.
sorted function
The sorted function Returns a sorted list of the elements of an iterable object. It is useful for sorting a list of numbers or strings, for example:
numbers = [3, 1, 4, 2, 5]
salary = sorted(numbers)
print(salary)
# Output: [1, 2, 3, 4, 5]
In this example, you can sort the list of numbers using the sorted function. The function returns a new list with the same elements, but in ascending order.
function reversed
reversed: Returns an iterable object with the elements of an iterable object in reverse order. It’s useful for reversing the order of elements in a list, for example:
numbers = [1, 2, 3, 4, 5]
reverse = reversed(numbers)
print(list(reverse))
# Output: [5, 4, 3, 2, 1]
In this example, we use the function reversed é to reverse the order of the elements in the number list. The function returns an iterable object with the elements in reverse order. Using the list function, we can convert the iterable object into a list and print the result.
The enumerate function
enumerate: Returns an iterable object that contains index and element pairs of an iterable object. It’s useful for iterating over a list and accessing both the index and the element on each iteration, for example:
fruits = ['apple', 'banana', 'orange']
for index, fruit in enumerate(frutas):
print(index, fruit)
# Output:
# 0 apple
# 1 banana
# 2 orange
In this example, we use the enumerate function to iterate over the list of fruits and access both the index and the element on each iteration. The function returns an iterable object that contains index and element pairs. Using a for loop, we can print the index and corresponding fruit on each iteration.
zip function
The zip function returns an iterable object that combines the elements of two or more iterable objects. It’s useful for combining two lists into a list of tuples, for example:
numbers = [1, 2, 3]
letters = ['a', 'b', 'c']
Combined = zip(numbers, letters)
print(list(Combined))
# Output: [(1, 'a'), (2, 'b'), (3, 'c')]
In this example, we use the zip function to combine the elements of the number and letter lists. The function returns an iterable object that contains tuples with one element from each list. Using the list function, we can convert the iterable object into a list and print the result.
function all
all: Returns True if all elements of an iterable object are true and False otherwise. It’s useful for checking whether all elements in a list are true, for example.
numbers = [1, 2, 3, 4, 5]
all_positives = all(number > 0 for number in numbers )
print(all_positives)
# Output: True
negative_numbers = [-1, -2, -3, 4, -5]
all_positives = all(number > 0 for numbers in negative_numbers)
print(all_positives)
# Output: False
In this example, we use the all is function to check whether all numbers in a list are positive. The first list only contains positive numbers, so the function returns True. The second list contains negative numbers, so the function returns False.
any functione
any: Returns True if at least one element of an iterable object is true and False otherwise. It’s useful to check if at least one element of a list is true, for example.
numbers = [1, 2, 3, 4, 5]
at_less_one_pair = any(numbers % 2 == 0 for numbers in numeros)
print(at_less_one_pair) # Output: True
odd_numbers = [1, 3, 5, 7, 9]
at_less_one_pair = any(numbers % 2 == 0 for numbers in odd_numbers)
print(at_less_one_pair)
# Output: Falsem
In this example, we use the any function to check if there is at least one even number in a list. In contrast, the first list contains at least one even number, so the function returns True. In contrast, the second list only contains odd numbers, so the function returns False.
For sure! In addition to the 10 functions we mentioned, there are many other functions in Python that are similar to the len function. Knowing these functions can help you write more efficient and readable code.
3 interesting curiosities of the len python function
Here we have 3 interesting facts about the len function in Python:
- The len function is a Python built-in function, which means that it is available in any Python program without the need to import external modules.
- In Python, we can use the len function on several types of objects, such as lists, tuples, strings, dictionaries, sets and other iterable objects.
- While most Python implementations of the len function have O(1) time complexity for fixed-size objects, some implementations may have O(n) time complexity for variable-size objects such as lists or dictionaries. This is because the len function needs to loop through all elements of the object to count the number of elements. Also, it’s important to note that other factors, such as the amount of memory available on the system, can affect the running time of the len function. On the other hand, it is important to highlight the performance of the len function when working with large or complex objects in Python.
Where often is the len function used in python?
In Python, the len function is often used to obtain the dimension of iterable objects, covering a variety of data structures such as lists, tuples, strings, dictionaries, sets, and many others. This function plays an essential role in providing information about the size of the elements contained in these objects. Several areas of software development make frequent use of the len function, including:
- A common way to perform element counting in various data structures is by using the len function. Extensive use of this function occurs for the purpose of establishing the count of the number of existing elements in various data structures, including lists, tuples, sets, strings and dictionaries. Thus, it plays a fundamental role in allowing the precise determination of the amount of elements present in each of these structures.
- One way to validate user input is through the len function, which allows you to check whether the input size is within the desired limits, either min or max. In this way, it is possible to ensure that the information entered by the user is in accordance with the specifications of the system or application in question.
A bit more about Python’s uses of len
When calculating indexes in Python, it is common to use the len function in conjunction with the indexes. This practice is especially useful for iterating over iterable objects such as lists and tuples. In this way, it is possible to obtain important information about the size and structure of these objects, allowing the programmer to develop more efficient and accurate solutions.
When we need to check whether two iterable objects have the same number of elements, we use the len function. This function is responsible for counting the number of elements present in each object and, thus, we can compare them to check if they are equal.
Calculating statistics involves using the len function to determine important measures, such as the mean, median, and mode, across different sets of data.
Conclusion
In Python, the len function plays a key role and finds application in many areas, from data manipulation to validating user input. Many people use it widely. We can see that it plays an important role in many tasks, making it one of the most used functions by programmers.
All in all, the len function is one of the most fundamental and useful functions in Python. It allows programmers to get the size of iterable objects such as lists, tuples, dictionaries, strings and sets. The len function not only counts the number of elements in an iterable object, it is also useful for validating user input, calculating statistics, and checking equality between iterable objects. It is noteworthy that the function is available in any Python program without the need to import external modules. Many different areas often, from manipulating data to getting information about the size of objects. Besides the ‘len’ function, another important function in Python is the ‘for’ function. To learn more about using the ‘for’ function, check out this article: For in Python: The indispensable function for efficient iterations.