String in Python: Creating, Formatting, and Manipulating Strings
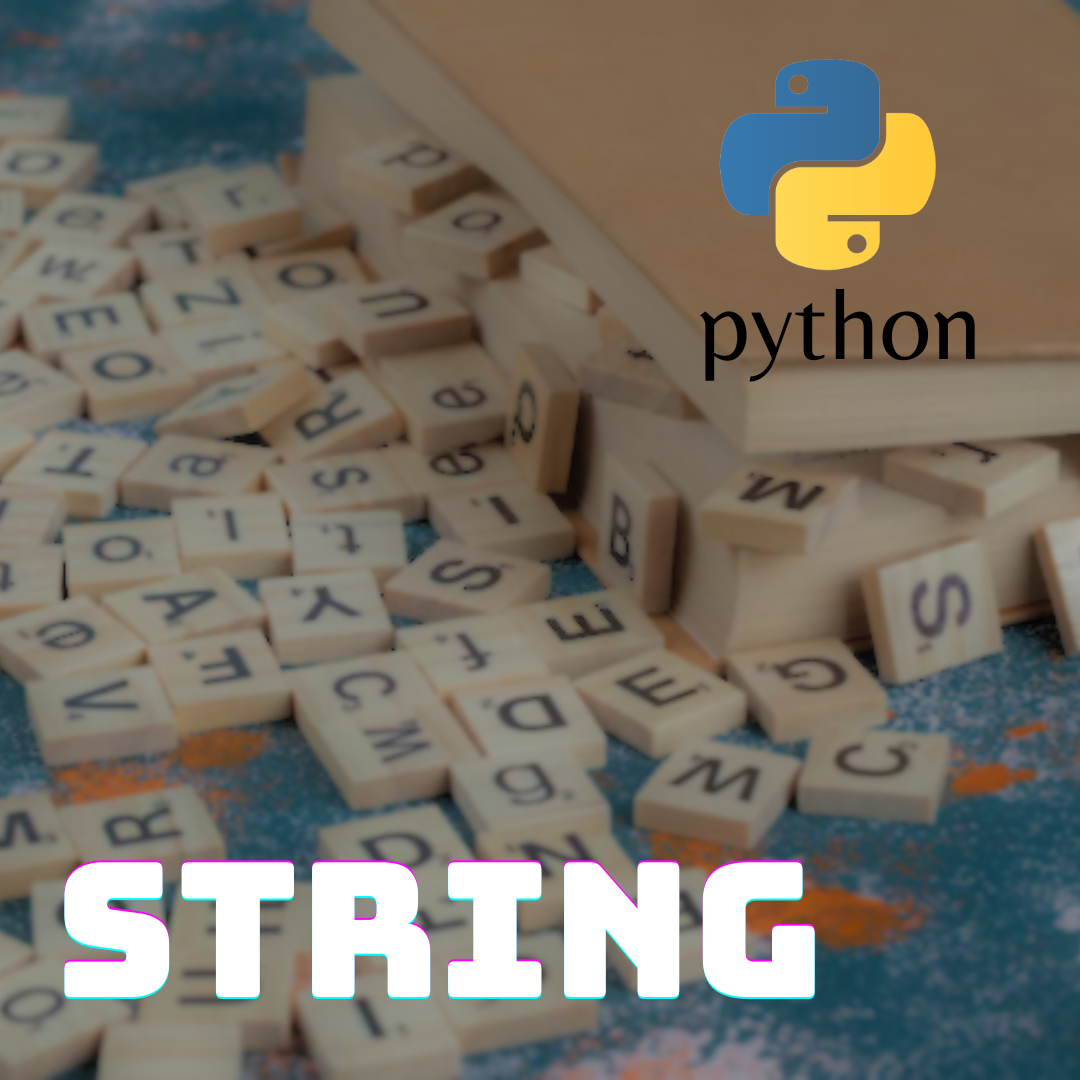
String in Python is a way of storing strings of characters, such as text, in a variable. In Python, we use the ‘ ‘ character (whitespace) to represent strings, which are immutable, meaning that their contents cannot be changed after creation. People use Python strings extensively in a variety of situations, ranging from manipulating human text to performing actions like replacing characters or concatenating strings.
In this article, we’ll explore the different aspects of strings in Python and see how they can be used to solve problems in various fields of application. Therefore, by the end of the article, you will have a deeper understanding of the different types of strings in Python, how to format strings and manipulate substrings, and you will be able to write your own code to manipulate strings efficiently.
Table of Contents
Common ways to work with strings in python
Here are some common ways to work with strings in Python:
- Creating strings: Strings can be created using the ‘ ‘ character (whitespace), such as “hello world” or using the str() method on objects, such as the string “hello world”.
- String formatting : Strings can be formatted using the + method or the format() method.
- String operators and attributes: Strings have various operators and attributes, such as concatenation, length, position, and built-in methods such as upper() and lower().
- String manipulation with built-in methods: Python has several built-in methods for string manipulation, such as split() for splitting strings into substrings, replace() for replacing substrings, and strip() for stripping leading and trailing whitespace. the end of a string.
- Concatenating strings with f-strings: As of Python 3.6, it is possible to use f-strings (format string literals) to concatenate strings and format numeric values and other data types directly inside strings, without needing format methods ().
- Types of Strings in Python: Python has three types of strings: strings of type str, strings of type unicode and strings of type bytes. The difference between these types is in the way they store characters in memory.
- String and memory: In Python, we represent strings in memory as a null-terminated sequence of characters. This means that to store a string in a variable, you must include a null character at the end of the string.
- String comparison with the ‘==’ method: to compare two strings in Python, we can use the == operator. We can also use the equal() method to compare two strings.
- Exploring the Python string library documentation: For more information on working with strings in Python, you can refer to the official Python string library documentation.
Creating string using ‘ ‘ character
In Python, a string is a type of variable that represents a sequence of characters. You can create a string using the space character, ” or ‘ ‘.
In the example below, we create the string “John Doe” using the space character. We use the space character to separate the words in the string. In Python, we represent the space character by ASCII 32 code. See:
name = "John Doe"
print(name)
Output:
John Doe
In another example, we create the string “John” using the space character. We use the space character to separate the username “John” from the string that fills with age. So we use the syntax f to insert the value of the variable “age” in the string. Look:
name = "John "
age = 30
print(f"Hello, my name is {name} and I am {age} years old.")
Output :
Hello, my name is John and I'm 30 years old.
In summary, in Python it is possible to create strings using the space character ‘ ‘. This is useful when you need to separate different elements in a string, such as separating the user’s name from their age.
Formatting strings with ‘+’ and format()
In Python, it is possible to format strings using the ‘+’ operator and the format() function.
We use the format() function to format strings with predefined values. It allows you to insert numerical values with two decimal places, numerical values with an exponent, strings, among others.
In this example, we use the format() function to insert the value of the variable “number” into the string. We use {} to allow insertion of the variable in the string. Look:
number = 123.456
name = "John Doe"
date = "2023-04-27"
print("The value is: {}".format(number))
Output:
The value is: 123.456
Another example, the format() function used to insert the value of the variable “date_birth” in the string. The format() function used to insert the string “years old” into the string. Look:
number = 123.456
birth_date = "1995-03-15"
print("I'm {} years old.".format(birth_date))
The output is:
I'm 1995-03-15 years old.
Furthermore, it is possible to format strings using the ‘+’ operator or the str.format() method. The ‘+’ operator lets you format strings with numeric values and simple strings, while the str.format() method lets you format strings with numeric values and more complex strings.
In the example, we used the format() function to insert the values of the variables “number” and “date” in the string. However, it is also possible to format the string using the ‘+’ operator as follows:
number = 123.456
date = "2023-04-27"
print("The value is": {} {}".format(number, date))
The output is:
The value is: 123.456 2023-04-27
In summary, in Python it is possible to format strings using the ‘+’ operator or the format() function. The ‘+’ operator is used to format strings with numeric values and simple strings, while the format() function is used to format strings with numeric values and more complex strings.
String operators and attributes
The String operators allow you to perform simple string operations such as concatenation, concatenate, cut and replace.
- Concatenate: Used to unite two or more strings.
string1 = "Hello"
string2 = "World"
string3 = string1 + " " + string2
print(string3)
The code returns:
Hello World
- Cut: We use it to remove a substring from a string.
string = "Hello World"
substring = string[:5]
print(substring)
The code returns:
Hello
- Replace: We use it to replace one part of a string with another.
string = "Hello World"
substring = "World"
new_string = string.replace(substring, "Python")
print(new_string)
The output will be::
Hello Python
In addition, strings also have some attributes that allow you to perform operations with them.
- Length: Used to get the number of characters in a string.
string = "Hello World"
print(len(string))
The return code will be:
13
- Slice: We use it to extract a part of a string.
string = "Hello World"
substring = slice(0:5)
print(substring)
The output will be:
Hello
- Upper: We use to convert all letters of a string to uppercase.
string = "hello world"
upper_string = string.upper()
print(upper_string)
The output will be:
HELLO WORLD
In summary, in Python it is possible to perform operations with strings using operators and attributes. String operators let you perform operations like concatenation, trim and replace, while string attributes let you get the number of characters in a string and extract a portion of a string.
tring concatenation with f-string
You can concatenate strings using the period character “.” or the “+” concatenation operator. However, with the introduction of f-strings (format string literals), string concatenation has become even simpler and more readable.
f-strings are a way to create concatenated strings from variables and values. They start with the letter “f” followed by a pair of square brackets “[” and end with a pair of square brackets “]”. So, inside these square brackets, we can specify the values we want to concatenate.
For example, suppose we want to concatenate a string with a name and an address. Using the f-string, we can write the f-string using the values of the variable “name” and “address” to form the concatenated string. Look:
name = "João"
address = "Road A, 123"
print(f"Hello, {name}! You live at {address}.")
The code output will be:
Hello, João! You live at Road A, 123.
Another advantage of f-strings is that you can use string formats to insert numeric or boolean values directly into the concatenated string. For example:
your_idade = 25
if_you_are_working = True
print(f"You are {your_age} years old and currently working.")
The code output will be:
You are 25 years old and currently working.
Thus, f-strings make string concatenation even simpler and more readable in Python.
String manipulation with built-in methods
There are several built-in methods you can use to manipulate strings. These methods help accomplish common tasks like adding, removing, or changing characters within a string. Some of the more common methods include:
upper()
It is lower()
These methods convert the string to uppercase or lowercase, respectively.
string = "Olá, mundo!"
string_uppercase = string.upper()
string_lowercase = string.lower()
print(string_uppercase) # Output: OLA, MUNDO!
print(string_lowercase) # Output: ola, mundo!
strip()
This method removes any whitespace on either side of the string.
string = " Hello World! "
stringa_no_space = string.strip()
print(string_no_space)
The output will be:
Hello World!
replace
()
This method replaces all occurrences of a specific substring with another one.
string = "Hello World!"
string_love = string.replace("hello", "love")
print(string_love)
The output will be:
love world!
split
()
This method splits a string into a list of substrings, separated by a specific substring.
string = "Hello, World!"
string_split = string.split(",")
print(string_split)
The output will be:
[‘Hello World!’]
join()
This method joins a list of strings into a single string, separated by a specific substring.
list_of_words = ["hello", "world!"]
string_join = " ".join(list_of_words)
print(string_join)
The output will be:
hello world!
These are just a few examples of the built-in methods available for manipulating strings in Python. There are many other methods and attributes available that we can use to perform a variety of tasks, such as formatting numbers, removing special characters, and much more.
With knowledge of these methods, you can manipulate strings more effectively and create more powerful and flexible Python programs. Furthermore, these methods are useful not only for string manipulation, but also for other general text processing tasks.
String types in Python (str, unicode, bytes)
There are three main types of strings: native strings (str) , Unicode strings (unicode) and byte strings . Below is an explanation of each:
- Native String (str): This is the default string in Python. It is composed of ASCII character and is suitable for representing plain text. The native string is represented as a sequence of characters.
- String Unicode (unicode): is a string that can contain characters from any language in the world. It is represented as a string of Unicode codes. In Python 2, strings were standard Unicode, but in Python 3, Unicode strings were replaced by byte strings.
- String bytes: is a string that contains binary data, such as an image or a file. A string in Python can be represented as a sequence of bytes. In Python 2, byte strings were the default string form, but in Python 3, byte strings were removed and the native string is now the standard string form.
In general, we use Unicode strings to represent text containing characters from different languages, while byte strings are used to display binary data. Choosing the appropriate string depends on the type of data you are working with and what you want to do with that data.
string and memory
Strings in Python are stored in memory, and the exact length of a string can vary depending on the size of the text or binary data it represents.
When we create a string, it is stored in memory as a sequence of characters or bytes, depending on the type of string we are using. The exact length of the string is related to the size of the text or binary data it represents.
For example, a simple string like “hello” only takes up a few bytes in memory, while a long string, like the full text of a book, requires many more bytes.
Also, in Python, strings are mutable, which implies that you can modify them after creation. This can affect memory as the string may need to be reallocated to accommodate the changes.
It’s important to remember that strings in Python are passed by reference, which means that when you pass a string to a function, you’re passing a copy of the string’s value, not a copy of the object itself. This can lead to unexpected behavior if you modify the original string within the function.
In short, Python strings are stored in memory, and the exact length of a string varies depending on the size of the text or binary data it represents. Strings are mutable and are passed by reference, which can affect memory if you modify the original string within a function.
String comparison in python with ‘==’ method
comparing strings with the ‘==’ method is an operation that checks whether two strings are equal. The ‘==’ operator compares the left string and the right string and returns True if they are equal and False if they are not.
For example, in the following code, we compare the two strings using the ‘==’ operator. As the two strings are the same, the program will display the message “Strings are equal” below:
# Create a string "Hello, World!"
string1 = "Hello, World!"
# Create other string "Hello, World!"
string2 = "Hello, World!"
# Comparing strings with the operator ==
if string1 == string2:
print("The strings are the same.")
else:
print("Strings are not equal.")
However, it is important to note that comparing strings with the ‘==’ method is sensitive to character order. Thus, this implies that the method considers the strings “abc” and “cba” as equal. If you want to compare strings in an order-insensitive way, use the .
Comparing strings with the ‘==’ method in Python is an operation that checks whether two strings are equal. However, we must be careful when using this method, as it is sensitive to character order.
Exploring the Python string library documentation.
The Python string library documentation is a very useful tool for learning about the different functions and methods available for working with strings. Here are some examples of how to explore the Python string library documentation:
- Open Python and type ” help(str) “. This will display documentation for the str class , which is the main class for strings in Python. You can browse this documentation and find information about different methods and attributes available for strings.
- Open Python and type “ help(string.upper) “. This will display the documentation for the upper() method of the str class. You can find information on how to use this method to convert a string to uppercase.
- Open Python and type “ help(string.join) “. This will display the documentation for the join() method of the str class. You can find information on how to use this method to join multiple strings into a single one.
- Open Python and type “ help(string.format )”. This will display the documentation for the format() method of the str class. You can find information on how to use this method to format strings with variable values.
In summary, the Python string library documentation is a valuable tool for learning about the different functions and methods available for working with strings. So, just type “help(function_name)” in Python to get information about the specific function. When studying string, it is also important to know about Switch Case , Scan , Len , While and For in python !