Python Scan: Complete Scan Function Guide
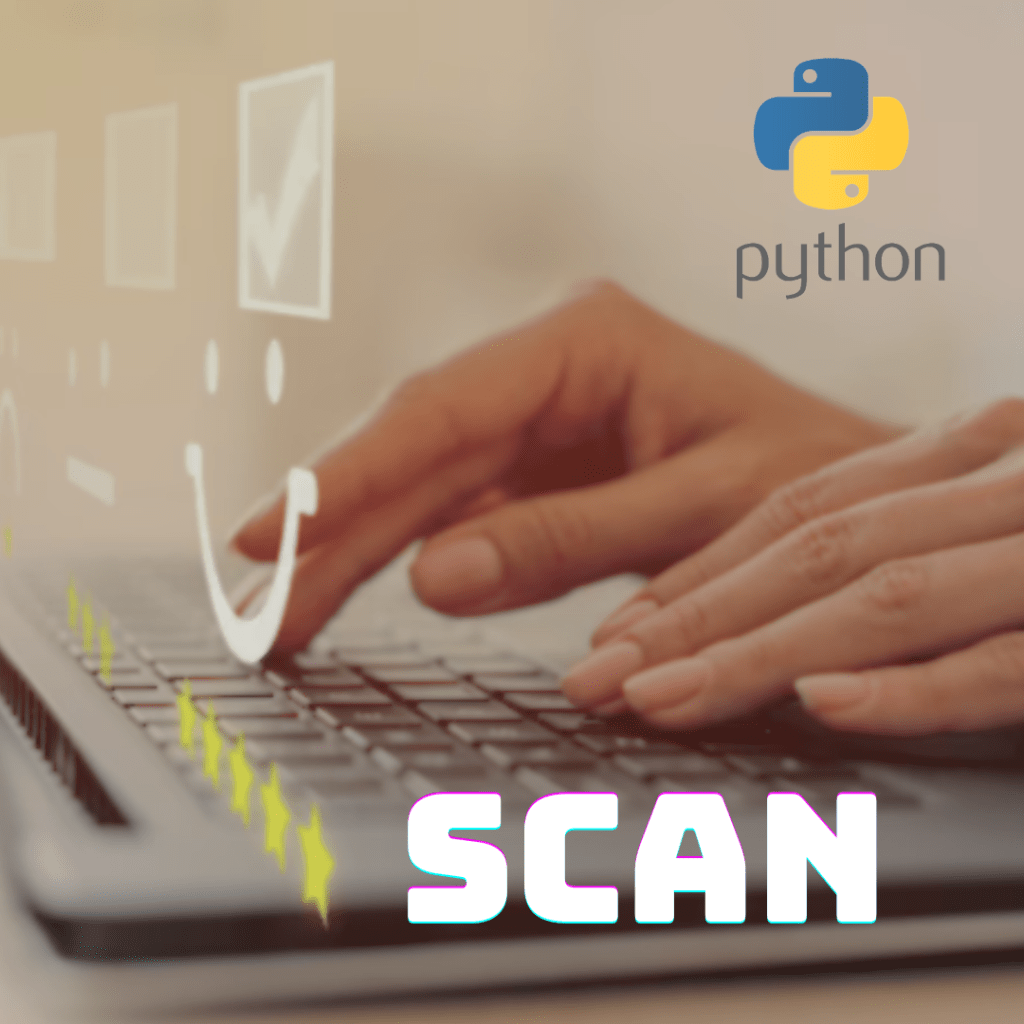
Scan in Python is a powerful tool for unit testing. It allows you to verify that a code is working correctly, identify potential problems and ensure that the code is operating as designed. With the use of scan in Python, it is possible to test a large number of cases in a short period of time, making the testing process more efficient. Also, what is python, you can easily use scan in Python and easily integrate it into your software development process.
In this article, you’ll learn about using scan in Python for efficient unit testing, its advantages, and how it compares to other Python unit testing methods. Take advantage of this opportunity to maximize the efficiency of your software development using Python scan.
[ez-tap]
What is scan in Python?
Python scan is a method used to analyze network traffic in real time. It consists of going through the information of a network in search of data packets that contain important information, such as IP addresses and ports. Scanning in Python can be done using libraries, such as Scapy, which provide tools for analyzing and manipulating network data packets.
Scan syntax in Python
Scan syntax in Python can vary depending on the library used. However, in general, it is necessary to import the scan library, such as Scapy, and create a function or program that traverses the network looking for data packets.
For example, the following code uses the Scapy library to scan a network for IP addresses and ports:
Import the Scapy library:
from scapy.all import *
Set the function to scan the network:
def scan(ip, port):
# Create a request packet (ARP) and send it to the specified port
arp_packet = ARP(pdst=ip)
arp_packet.send(port=port)
# Wait for the return of the reply packet (ARP)
response = arp_packet.recv()
# Display the results (address and port of the system that responded)
print(f"Address: {response.psrc}, Door: {response.dar}")
Specify the IP addresses and ports you want to scan:
scan("192.168.1.1", 80) # Example for port 80 from IP address 192.168.1.1
scan("192.168.1.1", 1234) # Example for port 1234 from IP address 192.168.1.
Advantages of using scan in Python
- Security analysis: You can use Python scan to identify security vulnerabilities in a network. It allows network administrators to be aware of the connections and communications taking place on their network, allowing them to take steps to secure it.
- Network monitoring: You can use Python scan to monitor activity on a network in real time. This allows network administrators to detect suspicious or abnormal activity and react quickly to mitigate it.
- Penetration Testing: It is possible to use the scan in Python to test the security of a network, identifying vulnerabilities and security flaws. This way, network administrators can take immediate action to fix these vulnerabilities before they are exploited by malicious attacks.
Examples of using scan in Python
1- Choose specific packages:
Python scan lets you choose which packages you want to scan. For example, one possible approach would be to specifically select Address Resolution Protocol (ARP) request packets, which may provide relevant information about IP addresses and ports. In this way, it is possible to obtain more specific data while scanning the network.
def scan(ip, port):
# Create an ARP request packet and send it to the specified port
arp_packet = ETHER(dst=ip)/ARP(pdst=ip)
arp_packet.send(port=port)
# Wait for return of ARP reply packet
response = arp_packet.recv()
# Display the results (address and port of the system that responded)
print(f"Address: {response.psrc}, Door: {response.dar}")
2 – Choose a specific network:
You can use Python scan to scan a specific network, such as a local network or a specific Wi-Fi network:
def scan(network_name):
# Create a Scapy program to scan the specific network
packet = IP(dst="ff02::1")/ICMP()
packet. spoof(dst="ff02::1", ipl=network_name)
packet.set_checksum()
packet.enable_checksum()
# Send packet and wait for response
response = sr1(packet, timeout=1)
# display the results
print(f"Address network IP: {response.src}, network mask address: {response.tgt}")
3 – Choose a specific protocol:
Instead of scanning all network connections, you can specifically choose a protocol, such as HyperText Transfer Protocol (HTTP), to analyze. For example:
def scan(port):
# Create an HTTP packet and send it to the specified port
packet = IP(dst="8.8.8.8")/TCP(dst="8.8.8.8", ports=str(port))/Raw(b"GET / HTTP/1.1\r\nHost: example.com\r\n\r\n")
# Send packet and wait for response
response = sr1(packet, timeout=1)
# display the results
print(response.text)
Application areas where scans are common in Python
Python is a programming language widely used in several areas, and the use of scans depends on the specific context of each application. Some areas where scans are common in Python include:
Network security
People widely use network scans to identify vulnerabilities in networks and systems. Below is example code using the nmap package to perform a port scan on a subset of IP addresses on a network. Look:
import nmap
subnet = "192.168.1.0/24"
scanner = nmap.PortScanner()
scanner.scan(subnet, arguments='-sS -T4')
for host in scanner.all_hosts():
print(host.address, host.port)
Pentesting
Pentesting is a common practice in software security and involves evaluating systems and applications to identify vulnerabilities and security risks. Below is a code example using the requests library to send POST requests with username and password data to a login URL. Look:
import requests
url = "http://example.com/login"
payload = {"username": "admin", "password": "password"}
response = requests.post(url, data=payload)
if response.status_code == 200:
print("Incorrect username and password!")
else:
print("Access denied!")
Data analysis
Scans are used to analyze large amounts of data in search of patterns, anomalies and other important information. Below is an example code using the pandas and numpy libraries to read and parse data contained in a CSV file. You can then use the scipy library to calculate the correlation between the data. Look:
import pandas as pd
import numpy as np
df = pd.read_csv("data.csv")
df.head()
# data normalization
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
df_scaled = scaler.fit_transform(df)
# data correlation
from scipy.stats import pearsonr
corr = pearsonr(df_scaled)
System monitoring
People use scans widely to monitor systems and applications in real time, identifying issues and inconsistencies. Below is a code example that uses the psutil library to monitor network traffic in real time. In addition, it continually updates network data and displays details such as the amount of bytes sent and received, along with the number of packets sent and received. Look:
from psutil import net_io_counters
while True:
# network data update
net_io_counters(pernic=True).sort_keys()
print("updated data:", net_io_counters.net_bytes_sent, net_io_counters.net_bytes_recv, net_io_counters.net_packets_sent, net_io_counters.net_packets_recv)
# wait for 1 second before updating again
time.sleep(1)
Network traffic analysis
Network traffic scans are used to analyze network traffic for anomalies and threats. Below is a code example using the requests library to send an HTTP request to a URL and the pyshark library to capture network traffic. It then parses the HTTP response and displays the status code and content of the response. Look:
import requests
import pyshark
# capture network traffic
sniffer = pyshark.sniff(filter="tcp", prn=lambda x: print(x.summary()))
# send HTTP request
response = requests.get("http://example.com")
# parse HTTP response
print(response.status_code)
print(response.content)
These are just some examples of using Scan in Python that can be used in different areas of applications. Python offers a variety of libraries and tools for analyzing network traffic, and you can adapt it to many different situations.
Recommendations for using scan in Python
Network and security scanning is an important practice for identifying vulnerabilities and threats in systems and networks. In Python, there are several scanning tools available that can help detect security issues. However, it is crucial to follow best practices to ensure that scans are performed safely and efficiently. Furthermore, by adopting these practices, you will be strengthening your protection against potential cyber threats.
In this article, we provide recommendations for using scanning in Python, including using scan-specific libraries, configuration management tools, log analysis, network monitoring, penetration testing, risk analysis, strong authentication, encryption, and monitoring. of malicious activity. By adopting these practices, we can help ensure that we scan safely and effectively, protecting against cyber threats.
Here are some recommendations for using scan in Python:
- Use specific scan libraries like Scapy, Nmap, or Python modules to access scan APIs like the requests library.
- Use configuration management tools such as Ansible, Puppet or Chef to automate the scan process and ensure the settings are correct.
- Use log analysis tools to collect and analyze system and application logs to identify vulnerabilities and security issues.
- Use network monitoring tools to monitor network activity in real time and identify vulnerabilities and suspicious activity.
- Use penetration testing tools (pen testing) to simulate attacks and assess the effectiveness of system security measures.
- Use risk analysis tools to assess risks associated with potential threats and vulnerabilities.
- Use strong authentication tools, such as two-factor, to secure access to critical systems and applications.
- Use encryption tools to protect data and communications.
- Leverage malicious activity monitoring tools to detect suspicious activity in real-time and quickly respond to threats.
- Use vulnerability analysis tools to identify and fix vulnerabilities in critical systems and applications.
Scan and other functions in python
The method scan()
can be used to perform specific tasks in conjunction with other Python functions to simplify the code and make it more readable and easier to understand.
In the example, it shows how the method can be used in conjunction with the functions Len in python
and For in Python
to accomplish a specific task.
The function add_sequences()
takes a sequence of values and uses the method scan()
to sum the values in the sequence until the counter reaches the length of the sequence. Then the function calls the function len()
to get the length of the sequence and uses a loop for
to count the number of elements in the sequence, which is passed as a parameter to the function add_sequences()
and finally, the code prints the result of the sum and the final counter.
def add_sequences(seq):
result = 0
counter = 0
max_len = len(seq)
while counter < max_len:
result += seq[counter]
counter += 1
return result
seq = [1, 2, 3, 4, 5, 6]
result= add_sequences(seq)
print(result) # Output: 15
counter = 0
for _ in range(len(seq)):
counter += 1
print(counter) # Output: 6
Comparison of Python scan with other Python unit testing methods
Scan is a popular Python unit testing method to check if a value is present in a list. Other Python unit testing methods are similar to Scan and can also test for value presence in a list.
The “in” method
One of the similar methods to scan is the “in” method. The “in” method is a unit test method that allows you to check whether a given substring (called a “search”) is present in a string. For example, the following code uses the “in” method to test whether the string “banana” contains the substring “na”:
string = "banana"
if "na" in string:
print("The string contains the substring 'na'")
else:
print("String does not contain substring 'na'")
The “count” method
The “count” method is a unit test method that allows you to count the number of occurrences of a given substring in a string. For example, the following code uses the “count” method,
to count how many times the string “banana” appears in the list “fruits”:
fruits = ["banana", "apple", "orange", "banana", "avocado"]
for i in range(len(fruits)):
if fruits[i] == "banana":
quantity_bananas = fruits.count("banana")
print("The list contains", quantity_bananas, "instances of 'banana'")
The “sorted” method
The “sorted” method is a sorting method that receives a list of values and returns a new list with sorted values. For example, the following code uses the “sorted” method to sort the list “fruits” in ascending order:
fruits = ["apple", "banana", "orange", "avocado"]
ordered_fruits= sorted(fruits )
print(ordered_fruits)
The “any” method
The “any” method is a condition checking method that returns “True” if at least one of the conditions is true, and “False” if all conditions are false. For example, the following code uses the “any” method to check whether the list “fruits” contains any fruit:
fruits = ["apple", "banana", "orange", "avocado"]
if any(fruit == "apple" for fruit in fruits ):
print("The list contains an apple")
else:
print("The list does not contain an apple")
The “all” method
The “all” method is a condition checking method that returns “True” if all conditions are true and “False” if any condition is false. For example, the following code uses the “all” method to check whether all fruits in “fruits” are sweet:
fruits = ["apple", "banana", "orange", "avocado"]
if all(fruit == "apple" for fruit in fruits ):
print("All fruits are apples")
else:
print("Not all fruits are apples")
In summary, the mentioned methods are similar to scan and you can use them to test if a given value is present in a list. Each method has its own advantages and disadvantages, and the choice of which method to use depends on the specific use case.
Conclusion
The Python scan is a powerful tool to identify vulnerabilities and threats in systems and networks. With the Python syntax, you can create custom scripts that automatically and efficiently perform scans. The advantage of using scan in Python is that you can perform real-time unit tests, detecting vulnerabilities before attackers exploit them.
There are many application areas where scans are common in Python, including network security, pentesting, data analysis, system monitoring, and network traffic analysis. However, it is important to remember that you should use scanning safely and with best practices in mind.
In this article, we present examples of using Python scan, compare Python scan to other Python unit testing methods, and provide recommendations for using Python scan. By adopting these practices, we can ensure that we scan safely and effectively, protecting against cyber threats. So, if you are looking for a powerful and flexible unit testing tool to identify vulnerabilities in your systems and networks, Python scan might be the right choice for you. In addition, this tool offers comprehensive analysis and detection capabilities, allowing you to thoroughly assess the security of your systems.