What is Python? Understand everything about this language.
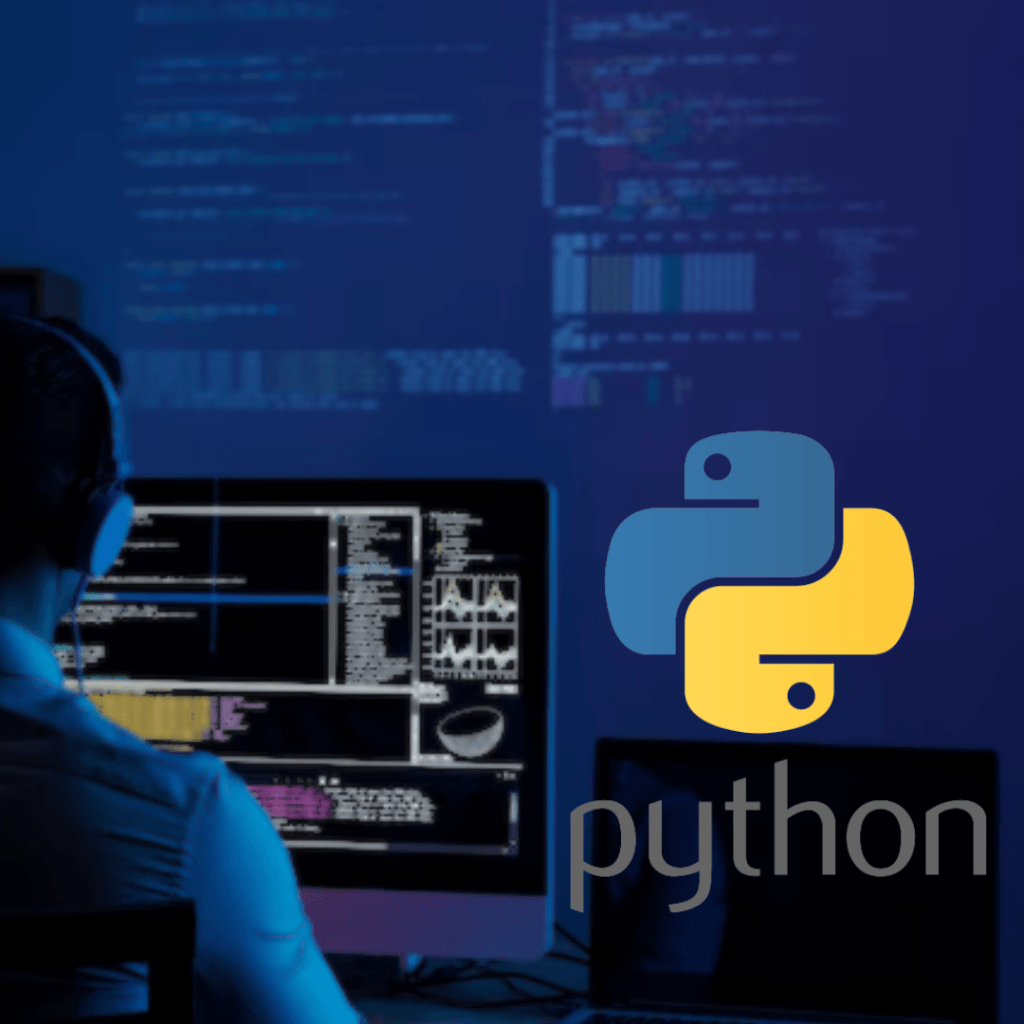
Python is a high-level, open-source, interpreted programming language that has become one of the most popular and widely used in software development. Founded in 1989 by Guido van Rossum, Python is known for its ease of learning, clear and concise syntax, and wide range of applications, including web development, data science, machine learning, automation of tasks, among others.
The language’s history began in 1989 when Guido van Rossum, a Dutch programmer, started working on a new programming language. He wanted to create a language that would be easy to learn and use, with clear and concise syntax, without sacrificing efficiency and scalability. He was inspired by other popular languages of the time, such as C, C++, Fortran, and Amoeba.
In this article, we will explore the language of Python even further, discussing its syntax, features, advantages, uses in different sectors, and future prospects. However, we will show how the language can help solve problems and create amazing applications, becoming a valuable tool for any developer or student interested in learning more about programming.
Table of Contents
Syntax of Python
Basic syntax of Python. Here are some key syntax concepts in basic Python:
Variables
Variables are used to store values. You can define a variable using the assignment operator (=). See an example:
x = 10
y = "hello"
Data types
There are many data types, including numbers, strings, lists, tuples, and dictionaries. So, see an example:
x = 10 # whole
y = "hello" # string
my_list = [1, 2, 3, 4] # list
my_tuple = (1, 2, 3) # tuple
my_dict = {"key": "value"} # dictionary
Operators
Operators are used to perform operations on values. In Python, there are several operators, including arithmetic operators (+, -, *, /), logical operators (and, or, not), comparison operators (==, !=, <, >, <=, >=) , between others. See an example:
x = 10
if x > 5:
print("x is greater than 5")
else:
print("x is less than or equal to 5")
while x > 0:
print(x)
x -= 1
for i in range(5):
print(i)
Functions
Functions are used to perform specific tasks, such as the len() function which is used to return the length of an object. In Python, you can define a function using the keyword def
. See an example:
def add_numbers(x, y):
return x + y
result = add_numbers(10, 3)
print(result) # print 13
Fundamental concepts of Python
Fundamental Python concepts include objects, classes, and modules. These concepts are important for understanding how Python works and how you can use the programming language to develop applications.
Objects
In Python, an object is an instance of a class. We use objects to store data and perform operations on that data. You can create an object using a keyword class
. In the example, the code defines a class “Person” with two attributes “name” and “age”, and a method “say_hello” that prints a message with information about the person. The class has a constructor that initializes attributes when creating an object. Look:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print("hello, my name is", self.name, "and I am", self.age, "years old")
person1 = Person("John", 30)
person1.say_hello() # print"hello, my name is John and I am 30 years old"
Classes
A class is a template for creating objects. Thus, we can use a class to define the structure and attributes of an object. You can define a class using a keyword class
. See an example:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
print("woof, woof")
dog1 = Dog("Buddy", 3)
dog1.bark() # print "woof, woof"
Modules
A module is a file that contains definitions of classes, functions and variables. So you can use modules to reuse code in multiple programs. There are two types of modules: standard modules, which come with the programming language, and third-party modules, which are created by other people. See an example:
import math
print(math.sqrt(16)) # print4.0
# loading a third-party module
import pandas as pd
data = pd.read_csv("data.csv")
print(data.head()) # print first records from csv file
Python libraries and frameworks
One of the main reasons Python is so popular is the amount of libraries and frameworks available to help developers build high-quality applications in less time and effort. We’ll introduce some of the most popular Python libraries and frameworks and explain how you can use them to develop Python applications.
NumPy
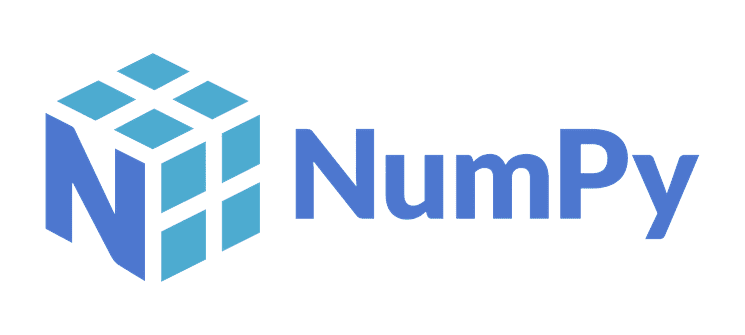
NumPy is a Python library that provides support for working with matrices and numerical arrays.
The library, moreover, is extremely useful for data processing tasks in Python. This is due to the fact that it makes it possible to work with large amounts of data quickly and efficiently. Additionally, some of NumPy’s most popular features include creating matrices, mathematical operations, and data truncation.
Pandas
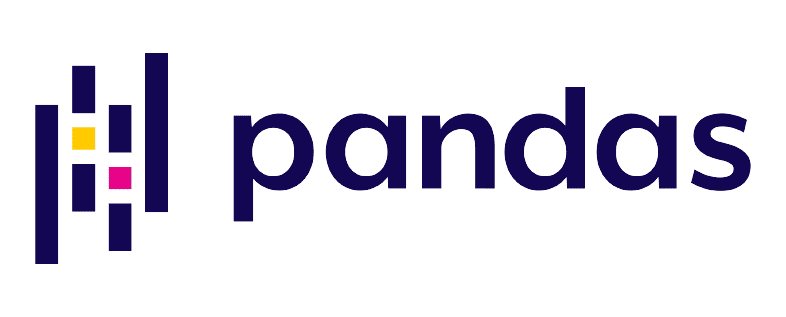
Pandas is a Python library that provides a flexible and easy-to-use data structure for manipulating and analyzing data. This library is very useful for data analysis tasks in Python, as it allows you to work with data in table format and perform operations such as aggregation, summarization and data cleaning. Some of Pandas’ most popular features include creating tables, removing duplicate values, and visualizing data.
Django
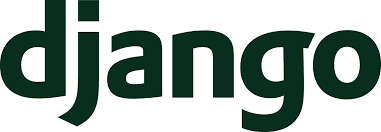
Django is a framework for web development. This library, moreover, is extremely useful for developing web applications in Python. This is because it offers a complete framework and, additionally, a series of tools to simplify the entire development process. Some of Django’s most popular features include creating data models, routing controls, and session management. Django is also known for its database management capabilities, which makes the process of creating Python web applications much easier.
Flask
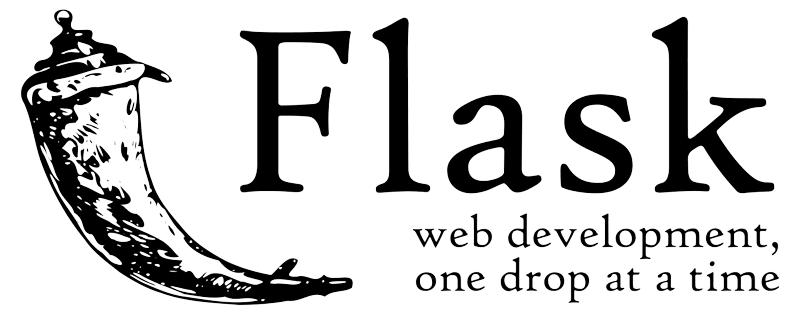
Flask is another Python framework for web development. However, it is a library used a lot to develop web applications in Python, as it provides a flexible and easy-to-use framework. Flask is especially useful for developing simple, lightweight web applications such as APIs or small applications. Some of Flask’s most popular features include route creation, session management, and integration with other popular Python libraries.
Python Applications
These are some applications of language use:
Web development
Python is one of the most popular languages for web application development, thanks to its libraries and frameworks such as Django and Flask. These tools allow developers to easily create high-quality web applications and are widely used to develop e-commerce applications, blogs and content management systems.
Data analysis
In data analysis we have libraries, such as NumPy and Pandas, that allow developers to process large amounts of data quickly and efficiently, and perform advanced data analysis. People widely use the Python language for data analysis tasks in areas such as data science, marketing, and finance.
Artificial intelligence
When we talk about artificial intelligence, the language is widely used, thanks to its libraries, such as TensorFlow and PyTorch. These tools allow developers to build machine learning models and other artificial intelligence technologies like speech recognition and computer vision.
Games
Python is one of the most popular programming languages for game development thanks to its libraries such as Pygame and PyOpenGL. In this way, tools allow developers to create games quickly and easily, and are widely used to create simple and complex games, such as platform games and strategy games.
Task automation
Python is one of the most popular programming languages for task automation, thanks to its libraries such as Selenium and PyAutoGUI. However, these tools allow developers to create task automation applications such as automating software tests and creating virtual assistants.
How to start programming?
So to get started programming in Python, follow these tips and resources:
Install
- Download and install the latest language version from the official website.
- Make sure you install correctly and make it clear which version is installed (see an example, Python 3.x)
Online tutorials and courses
- Codecademy: Codecademy offers a free course that includes hands-on exercises and small projects to help consolidate knowledge.
- Coursera: Coursera has several free and paid courses on the language, including Intro to Programming from the University of Michigan.
- Real Python: The site offers a variety of free tutorials and courses, including Introduction to the Language for Computer and Web Scientists.
official documentation
- Official Python documentation: The documentation includes sections on syntax, semantics, standard modules, and other Python functionality.
Discussion sites and forums:
- Reddit: The r/learnpython subreddit is indeed a popular place for Python questions and answers. You can find solutions to specific problems, programming tips, and much more.
- Stack Overflow: Stack Overflow is a popular Q&A platform for programmers. Therefore, you can find and answer questions about Python, as well as consolidate your knowledge by reading and answering questions from other users.
- Python.org: The official site has a forum section where you can find answers to frequently asked questions, share code, and discuss Python-related topics.
future of language
It is a high-level programming language that has experienced exponential growth over the past few decades, yet it is expected to continue to be one of the most popular languages in the future. Some of Python’s trends and futures include:
Adoption in companies
Companies around the world are increasingly adopting Python, one of the most popular languages for software development. It is an easy-to-learn, flexible and highly scalable language, which makes it ideal for large or small projects. Furthermore, it is worth noting that there is also a wide variety of Python libraries and frameworks available, which play a key role in accelerating development and increasing productivity.
Development of new libraries and frameworks
Python has a large community of developers who regularly contribute to the language ecosystem. This means that new Python libraries and frameworks are constantly being created to meet the needs of developers. Indeed, some examples include TensorFlow and PyTorch for machine learning, Django for web application development, and Pandas and NumPy for data analysis.
Future language updates
Although the language has a clear and concise syntax, it has some limitations that future updates may fix. For example, support for static types can be added to improve security and code speed. Thus, the Python community is working on updates to improve the performance of the language in large-scale applications.
In summary, it has a promising future as a popular and highly used programming language around the world. Enterprises will continue to adopt and developers will create new Python libraries and frameworks to meet their needs. And so, it’s important to point out that future language updates may help to correct some of the current limitations and improve performance in large-scale applications. In this way, the language will continue to be a crucial and versatile programming language for developers all over the world.