Input python: How to validate and manipulate user data
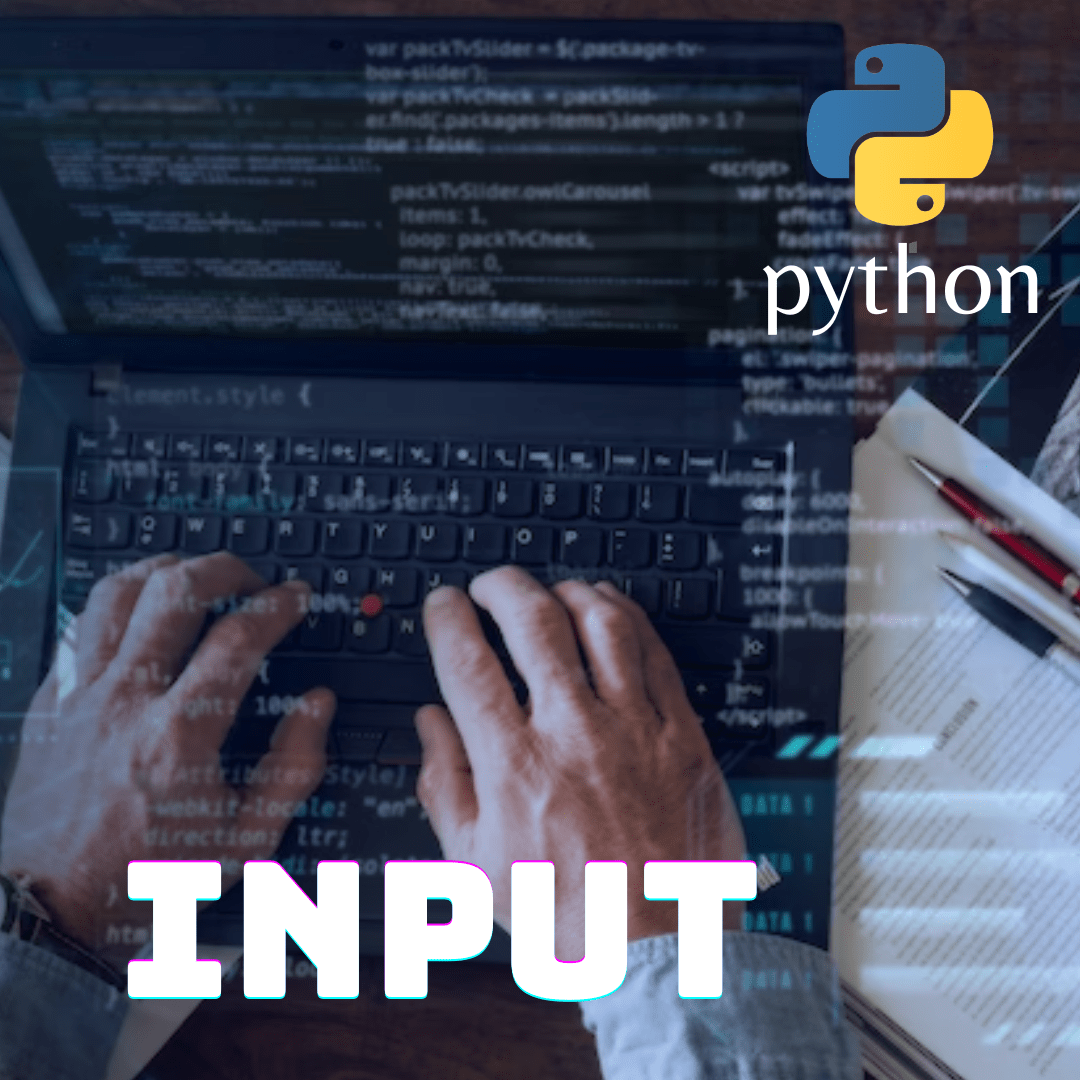
Inputting data is a fundamental part of any program, and the input function in Python provides an easy way to get input from the user . Thus, this function allows the programmer to ask the user to enter an input, which can be a string , an integer number, decimal or a string with numerical digits, among other types of data.
The ‘input()’ function is one of the most commonly used functions in Python, as it is an easy way to get information from the user to use in a variety of programs, from simple scripts to complex applications. In addition, Python offers a variety of ways to get user data, including reading from files and manipulating data from external sources such as APIs and databases.
So this article will explore how to use the ‘input()’ function in Python , including the different types of input you can collect and how to validate and manipulate this data for use in your programs. In addition, we’ll discuss advanced Python input techniques, such as using regular expressions for input validation and accessibility of input data in dictionaries.
Table of Contents
Input function syntax in Python
The Python function input()
is used to get a line input from the user’s screen. The basic syntax is as follows:
response = input("search for something: ")
Where “Search for something” is a string shown on the user’s screen to type the answer. Thus, function input()
returns the string typed by the user and stores it in the variable resposta
.
You can also specify a format for the input, such as an integer, using the int(resposta)
or syntax float(resposta)
. For example:
number = int(input("Enter an integer: "))
Or
can = float(input("Enter a decimal position: "))
Also, the function input()
returns an empty string ( ""
) if there is no input provided by the user. You can use the expression if resposta == ""
to check whether the input was provided or not.
response = input("search for something: ")
if response == "":
print("No input provided")
else:
print("Response:", response)
Input Validation in Python
Input validation in Python is an important technique for ensuring that your program receives valid, human-readable input. This is especially important when the program is intended for end users to use, as user input is unpredictable and erroneous.
There are many ways to validate input in Python, depending on the type of input and the needs of the program. Some of the more common techniques include:
Input validation using functions
We can create functions that check if a user’s input is valid or not. For example, to validate an integer input, we can create a function that checks if the number is a positive integer:
def is_positive_integer(x):
return x > 0
number = int(input("Enter an integer: "))
if is_positive_integer(number):
print(f"{number} is a positive integer!")
else:
print(f"{number} is not a positive integer!")
Input validation using regular expressions
Regular expressions are a powerful tool for validating input in Python. In this way, you can use a regular expression to check whether a data entry meets a specific pattern. For example, to validate a string input that contains only letters and numbers, we can use the regular expression ^[a-zA-Z0-9]+$
:
input_1 = input("Please enter a valid string: ")
if re.match(r"^[a-zA-Z0-9]+$", input_1 ):
print("Entry is valid!")
else:
print("Entry is not valid!")
Input validation using the module bool
The module bool
includes functions that can be used to validate input in Python. For example, the function bool()
can be used to check whether an input is a valid string or not:
input_2 = input("Please enter a valid string: ")
if bool(input_2 ):
print("Entry is valid!")
else:
print("Entry is not valid!")
In addition, the module bool
also includes the function not_
, which is useful for validating whether an input is false. For example, if you want to validate whether a user has entered an integer, you can check whether the function’s result int()
is false:
number = int(input("Enter an integer: "))
if not int(number):
print("This is not a whole number!")
Input validation using the module json
The module json
includes functions that can be used to validate input data in JSON format. For example, you can use the function json.loads()
to check if an input is a valid JSON object:
json_string = input("Please enter a valid JSON string: ")
if json.loads(json_string):
print("Entry is valid!")
else:
print("Entry is not valid!")
In summary, there are many ways to validate input in Python, and which technique is best for you depends on the type of input and the needs of the program. However, regardless of the technique chosen, it is important to ensure that the program receives valid and readable input data to avoid problems with the program’s operation.
Accessing input value in a dictionary in Python
In Python, it is possible to store user input values in a dictionary. This can be useful in situations where we need to store a series of input values in an organized format.
To access the input value of a dictionary, we make use of the syntax dictionary[key]
, where chave
is the key corresponding to the desired input value. For example:
words= {}
words["sentence1"] = input("type a sentence: ")
words["sentence2"] = input("type another sentence: ")
print("The sentence entered is:", palavras["sentence1"])
print("The sentence entered is:", palavras["sentence2"])
In this example, we are creating an empty dictionary called palavras
. Next we are adding two keys sentence1
and sentence2
with the user input values. Next, we’re accessing the sentence1
e key values sentence2
and displaying them on the screen.
Also, you can access an input value directly from the dictionary without assigning it to a variable. In this example, we are directly accessing the user input values directly from the dictionary palavras
, see:
palavras = {}
palavras["sentence1"] = input("type a sentence: ")
palavras["sentence2"] = input("type another sentence: ")
print("The sentence entered is:", palavras["sentence1"])
print("The sentence entered is:", palavras["sentence2"])
Usage examples for simple programs
There are many ways to use function input
in Python to collect user data and use that data in a program. So, here are some examples:
- Collect an integer from the user:
number = input("Enter an integer: ")
print("The number you entered is", number)
- Collect a string from the user:
text = input("Enter an string: ")
print("The string you entered is", text)
- Collect two strings from the user and concatenate them:
name = input("type your name: ")
surname = input("enter your last name: ")
print("Your first and last name are", name + " " + surname)
- Collect an integer and display a message if that number is even or odd:
number = input("Enter an integer: ")
if number % 2 == 0:
print("The number you entered is even")
else:
print("The number you entered is odd")
- Collect a string and loop through all the letters and add a comma after the first letter of each word:
text = input("type a string: ")
response = ""
for letter in text:
if letter.isalpha():
response += letter + "."
else:
response += letter
print("The string with the first letters of each word and a comma after them is: ", response)
These examples are just a small sample of how input
can be used in conjunction with other Python functions, such as for
, len
, if-else
, while
, to collect user data and do some operations with that data.
Input types in Python
Python provides several types of input to programs, including:
- By keyboard : With the ‘input()’ function we can use it to collect data from the user through the keyboard.
- File : Python lets you read data from files, such as text and numbers, and use that data in programs.
- URL : We can use it to read data from external sources, such as URLs, and use that information in programs.
- Streams : Python also lets you read data from streamed input sources, such as serial input devices, and use that information in programs.
- Command line input : Python allows you to read command line data, such as command line arguments passed to the program.
- Input of a function : Python allows a function to return input data as arguments.
So these are some of the input types available in Python, and each of them has its own advantages and specific uses.
Advanced input techniques in Python
There are several advanced Python input techniques that can be useful in different situations. Here are some of those techniques:
- Lecture: We can use this technique to read data from a file input source, user-written input, or data from an API.
csv
Python module is useful to read data from CSV file. The moduleinput
can also be used to read user input. - Entry: We can use this technique to insert data into a table or database. The
sqlite3
Python module is useful for inserting data into an SQLite database. The modulepsycopg2
is useful for inserting data into a PostgreSQL database. - Extract: We can use this technique to extract information from a file or data from an API. The
pandas
Python module is useful for extracting information from a CSV file. The modulerequests
can be used to make HTTP requests and extract information from an API. - Analyze: We can use this technique to analyze data. The
pandas
Python module is useful for performing data analysis operations. The modulenumpy
is useful for performing mathematical calculations on vectors and matrices. - Sort: We can use this technique to sort data. The
pandas
Python module is useful for sorting data based on one or more columns. - Filter: We can use this technique to filter data. The
pandas
Python module is useful for filtering data based on one or more columns. - Join: We use the
pandas
Python module that is useful for joining sets of data based on one or more columns.
These are just some of the advanced Python input techniques. Each technique has its own advantages and disadvantages, and choosing the right technique depends on the specific dataset and problem at hand.
mportant considerations when using input in Python programs
When using function input()
in Python programs, it is important to consider some important issues to ensure that the code works correctly and is secure. Here are some of those considerations:
1. Entry validation
In this regard, it is important to validate user input to ensure that it is of the expected type and format. In this way, we can use regular expressions or checking the data type. For example, if we expect the user to enter an integer, you can check if the input is an integer using the int()
.
2. Length limitation
If you’re asking the user to enter a string, it’s important to limit the length of the string to avoid buffer overflow. This way, you can use the function len()
to get the length of the string and check if it is less than a specific threshold.
3. Separation of input and output
It is important to separate user input from program output so that the user can interact with the program clearly. You can do this by creating a separate function for the input and another function for the output.
4. Security
It is important to prevent the user from sending malicious code or explanations to the program. Therefore, you can do this using techniques such as input validation and length limiting. It is also important to avoid executing user code, for example by avoiding the use of functions like eval()
and exec()
.
5. Typo error
It is important to deal with typos such as typing an invalid number or an empty string. Thus, this can be done using an exception such as ValueError
, to catch the error and display an error message to the user.
As such, when using function input()
in Python programs, it is important to consider issues of input validation, length limitation, separation of input and output, security, and typos to ensure that the code works correctly and is safe.
Conclusion
In conclusion, Python input is a fundamental skill for any Python developer. The function input()
is a powerful tool for collecting data from user input, but it’s important to consider input validation issues, length limitation, input-output separation, security, and typos to ensure that the code works correctly and is safe. With a good understanding of how input works in Python, you can create more efficient and robust programs to handle user input.