Append python: Learn to add elements efficiently
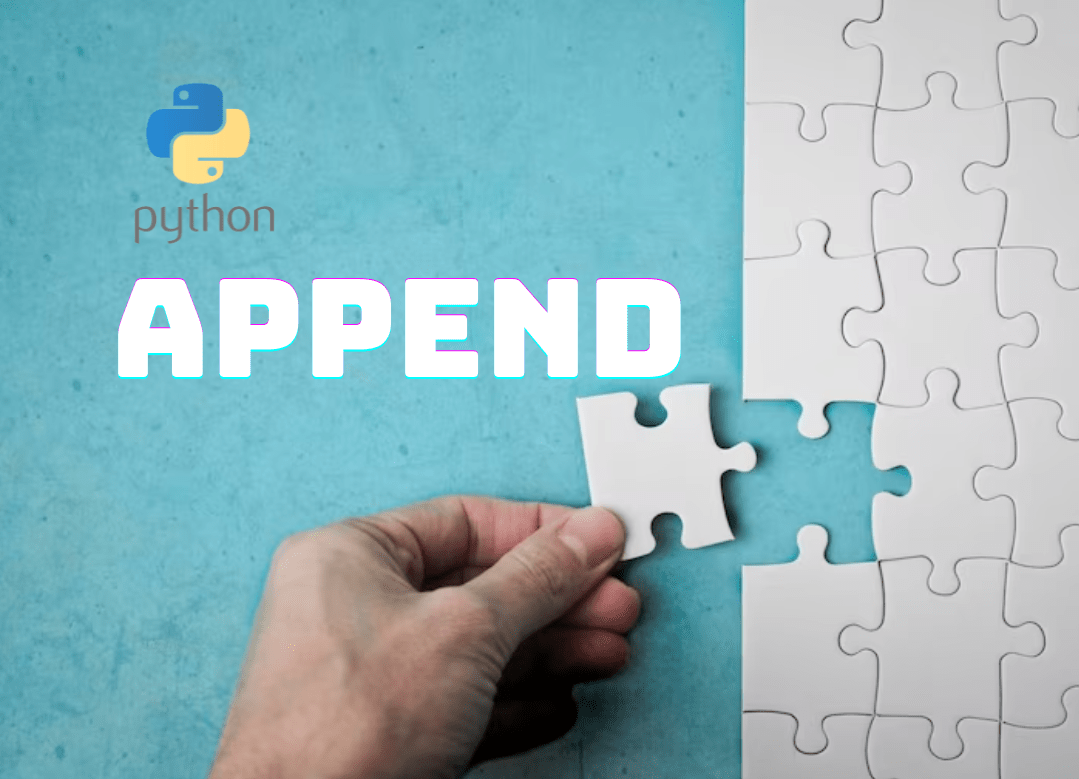
Python append module is a module that lets you add content to an existing list/array. It is very useful when you need to add information to a list without interrupting the flow of other operations on the file. With the append module, you can add lines of text, bytes, or other types of data to a file efficiently and securely.
In this article, we’ll explore the features and how to use Python’s append module in different situations.
Table of Contents
Append module syntax
Python’s append module syntax is simple and easy to learn. To add content to an existing file, you can use the “ append ” function of the append module. To use the “append” function is as follows:
list.append(element)
Where list
is the list or string to which you want to add an element and elemento
is the element to be added.
For example, suppose we have a list of integers called numbers
:
numbers = [1, 2, 3, 4, 5]
We can add a new integer to the end of the list using append
:
numbers.append(6)
Now the list number
will have the values:
[1, 2, 3, 4, 5, 6]
On the other hand, if we want to add an element to the end of a string, we can use the append
string method:
text = "Python is a programming language"
text.append(" very popular.")
Now the string text
will be:
Python is a very popular programming language.
Note that when using append
with strings, the new element will be added to the end of the string as a substring instead of creating a new string.
In summary, append
is a powerful tool for adding elements to the end of lists or strings in Python. The basic syntax is easy to understand and we can use it to efficiently and readably add elements to our code.
Examples of how to use append module in python in different situations
Here are some examples of how to use the module append
in different situations:
Adding a Single Item to a File
In this example code opens the file file.txt
in “add” mode ( 'a'
), which allows adding data to the end of the file. It then uses the append
object’s method f
to add the item Item to be added to the end
of the file.
with open('file.txt', 'a') as f:
f.append('Item to be added')
Putting multiple items to one file
Note that in this code we opened file.txt
using “add” ( 'a'
), as in the previous example. It then uses the append
object’s method f
to add multiple items to the end of the file, separated by a line break ( \n
).
with open('file.txt', 'a') as f:
f.append('\nItem 1')
f.append('\nItem 2')
f.append('\nItem 3')
Imputing data to a file that does not exist
Here we have some code that opens the file file.txt
in “add” mode ( 'a'
), as in the previous examples. However, if the file does not exist, Python will create a new file with the specified name.
with open('file.txt', 'a') as f:
f.append('Item to be added')
Append data to an existing file
we have in this example file.txt
that is open and “add” ( 'a'
), as in the previous examples. If the file already exists, the append
object’s method f
will add the data to the end of the file. Thus, if the file does not exist, Python will create a new file with the specified name.
with open('file.txt', 'a') as f:
f.append('Item to be added')
It is important to note that using the module append
must be combined with finalizing the file with the close
object’s method f
. This ensures that we write the file correctly and avoid errors in data handling.
Advanced append examples in python
The python append module can be used with other common features like for , null and switch case .
One of the ways to implement a “null” in Python is using the value None
. For example, if you wanted to add a line of content to the end of a file only if a specific value is None
, you could use the following code:
with open('file.txt', 'a') as f:
content = input('Enter the content to be added: ')
if content is None:
f.append('not added')
else:
f.append(content)
Here is the code that prompts the user to enter content to add to the file. If the entered value is None
, the “Not Added” content will be added to the file. Otherwise, the content you entered will be added to the end of the file.
Another way to implement a “switch case” in Python is:
If you want to add different values to the end of a file based on a specific value, you can use the following code:
with open('file.txt', 'a') as f:
for line in f:
value = line.strip()
if value == 'value1':
f.append('value1')
elif value == 'value2':
f.append('value2')
else:
f.append('value3')
So the code loops through each line of the file and removes whitespace using the strip
. It then compares the row value with ‘value1’, ‘value2’ and ‘value3’. If the value is equal to ‘value1’, we will add the content “value1” to the end of the file. This way, if the value is equal to ‘value2’, we will add the content “value2”. Otherwise, we will add the content “valor3”.
Benefits and challenges of using the append module
Python’s module append
is a convenient, shorthand way to add an element to the end of an existing list. Thus, it is especially useful when working with lists of variable length and when you want to add elements only to the end of the list, without having to reallocate memory or create a new list.
Key benefits of using the append module include:
- Memory saving: by adding an element to the end of an existing list, the module
append
avoids the need to create a new list, which saves memory space. - Flexibility: The module
append
allows adding elements to a list dynamically, which is useful in situations where the size of the list can vary. - Readability: Using the module
append
makes code more readable and concise, particularly in situations where you want to add multiple elements to the end of a list.
However, there are some challenges associated with using the append module in python, including:
- Complexity: The module
append
can make code more complex and harder to understand, especially for newer Python users. - Performance: Excessive use of the module
append
can affect program performance, especially in situations where you need to add many elements to a list. - Incompatibility: The module
append
is not compatible with all Python lists. For example, it cannot be used with empty lists or fixed-length lists.
In short, append
it’s a powerful and useful tool for adding elements to an existing list in Python, but it should be used with care and in appropriate situations to avoid performance issues and incompatibility.
Alternatives to append module in python
While modulus append
is a convenient way to add elements to the end of a list or string in Python, there are other ways to do this. Some of these alternatives include:
- List concatenation: We can add an element to an existing list using list concatenation. For this, we can create a new list that will contain the element we want to add and then concatenate this list with the existing list using the operator
+
. For example:
list = [1, 2, 3]
new_list = list + [4]
return, the variable new_list
with the values:
[1, 2, 3, 4]
- Method
extend
: The methodextend
can be used to add multiple elements to the end of an existing list. This way it accepts one or more arguments and adds them to the list. For example:
list = [1, 2, 3]
list.extend([4, 5, 6])
Now the list list
will have the values:
[1, 2, 3, 4, 5, 6]
- String concatenation: We can add an element to the end of a string using string concatenation. For that, we can use the operator
+
to concatenate the existing string with the new element. For example:
text = "Python is a programming language"
new_text = text + " very popular."
Thus, the variable new_text
will have the values:
Python is a very popular programming language.
- Method
join
: methodjoin
can be used to add an element to the end of a string using a delimiter. It accepts a delimiter and adds one or more arguments to the string. For example:
text = "Python is a programming language"
text = text.join(", ")
The output will be:
Python is a programming language,
In summary, there are several alternatives to module append
in Python for adding elements to the end of lists or strings. Each of these alternatives has its own advantages and disadvantages, and choosing the best alternative depends on the context in which we are working.
Features and usage of python append module
Python’s append module is a standard module that lets you add content to an existing file efficiently and securely. It has some important features that make it a valuable tool for manipulating files in programming projects.
One of the most important features of the append module is the ability to add content to an existing file. This means you can add new lines of text, bytes, or other types of data to a file without having to first create a new file or delete existing content. This is useful in situations where you need to add information to a file without interrupting the flow of other operations on the file.
Another important feature of the append module is the ability to remove lines from a file. This can be useful in situations where you need a cleaner, more organized file, or where you need to remove incorrect or unnecessary information.
The append module also has functions that let you add headers or footers to a file, and do other useful operations. In addition, it has file manipulation functions, such as opening, closing, and listing files.
To use the append module, you need to import it into your Python code. You can then use the functions available in the module to add content to a file, remove lines from a file, or perform other useful operations. The append module is easy to use and well documented, making it an ideal choice for anyone looking for ease and efficiency in file manipulation in Python programming projects.
Conclusion
In conclusion, Python’s append module is a valuable tool for manipulating files in programming projects. It offers a variety of features, including adding content to an existing file, removing lines from a file, adding headers or footers to a file, and many other useful operations. In addition, the append module is easy to use and comes pre-installed with Python, making it an ideal choice for anyone looking for ease and efficiency in file manipulation. So, with this module, we can perform a variety of file-related tasks in a simple and efficient way, which makes handling files in programming projects with Python easier and more productive.