init python: Learn how to use __init__ to initialize objects in python

Python init method is a special function that we can apply to initialize a class. When we create an object of the class, the method is automatically applied and defines the object’s initial behavior. In this sense, we can use the class object as a pointer to the object being initialized, accessing the class’s attributes and methods, and performing any other operations necessary to initialize the object.
In this article, we’ll explore the method __init__ in Python. Thus, learning about how we can use it to initialize classes, including what it is and how it can be applied to maintain data integrity. We’ll discuss how to create a method __init__ and the syntax needed to make it work. In addition, also learn about how to add elements efficiently and use both knowledge to improve your code.
Table of Contents
Syntax
We use the method __init__
in Python to initialize a class. When we input an object of the class, the method is applied automatically. Thus, the basic syntax of the method __init__
is as follows:
def __init__(self, *args, **kwargs):
# código a ser executado durante a inicialização do objeto
The first parameter of the method __init__
is the argument list ( *args
). We can use it to pass construction arguments to the class when we create an object. Also, if there are no construction arguments, we can pass None
to the first parameter.
The second parameter of the method __init__
is the initialization parameter list ( **kwargs
). We can be using it to pass initialization parameters to the class when we input an object. So the initialization parameters are being passed as an associative key-value where the key is the parameter name and the value is the parameter value.
We run the code inside the method __init__
when we create an object. In this way, we can use the class object as a pointer to the object being initialized, accessing the class’s attributes and methods, and performing any other operation necessary to initialize the object.
In this example below, we create a class Car
with a method __init__
that takes three parameters ( make
, model
and year
). Code within the method __init__
assigns these values to the corresponding attributes. So we also create a method start
and a method stop
that print information about the car. When we create an object of the class Car
and call the start
and methods stop
, the code inside the method __init__
is executed. Look:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def start(self):
print(f"Starting {self.make} {self.model} {self.year}")
def stop(self):
print(f"Stopping {self.make} {self.model} {self.year}")
car = Car("Toyota", "Corolla", 2022)
Examples of using the method __init__
on different types of objects in Python
The method __init__
is a special method in Python that is called automatically when we create an object. This way, the method can be used to initialize the object and assign values to its attributes. Here are some examples of how the init method can be used on different types of objects in Python:
1. Simple class objects:
We have a basic example of creating a Python class called “Person”. The class has a special method called “ init ” which is being called when we create an object of the class. This way, the method receives two arguments, “name” and “age”, which can be assigned to the attributes “name” and “age” of the Person object. In the end, we create a Person object receiving “Maria” and age of 25 and its attributes will be displayed in the console. Look:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
Person1 = Person("Maria", 25)
print(Person1.name) # "Maria"
print(Person1.age) # 25
2. Objects of more complex classes:
The class defines a constructor ( init ) to define the attributes of the class, which in this case are: make, model, year and price of the car. Next, we create an object of type “Car” and assign it the name “car1”. Finally, the attribute values of the object “car1” can be displayed in the console using the syntax “car1.attribute”, where the desired attribute name assumes “attribute”. Look:
class Car:
def __init__(self, brand, model, ano, preco):
self.brand = brand
self.model = model
self.year = year
self.price = price
car1 = Car("Ford", "Fiesta", 2015, 15000)
print(car1.brand) # "Ford"
print(car1.model) # "Fiesta"
print(car1.year) # 2015
print(car1.price) # 15000
3. List objects:
Now we have an example that creates a list of three elements: “Maria”, 25 and “Sao Paulo”. It then uses a loop for
to iterate over each element in the list and prints out each element. The syntax for elemento in lista
means that the variable elemento
will be updated on each iteration of the for loop , matching each element in the list. Thus, the loop prints each value of elemento
using the function print()
list1 = ["Maria", 25, "Sao Paulo"]
for element in list1:
print(element)
# Output:
# Maria
# 25
# Sao Paulo
4. Dictionary objects:
This example creates a Python dictionary with three keys and associated values: “name” with the value “Maria”, “age” with the value 25, and “city” with the value “São Paulo”. In this way, a loop for
is used to iterate over the dictionary items, which are the associated keys and values. The syntax means that the variable will be updated on each iteration, matching the dictionary key, and the variable will be updated with the corresponding key value. The loop prints each key and value using the . The output of this example will be “name”, “25” and “city”.for chave, valor in dicionario1.items() chavevalor print()
dictionary1 = {"name": "Maria", "age": 25, "city": "Sao Paulo"}
for key, value in dictionary1.items():
print(key) # "nome"
print(value) # 25
# Output:
# nome
# 25
# cidade
5. Custom class objects:
And finally, we have the following example creating an “Animal” class that has a constructor to define the name and age of the animal.
The class also has a “speak” method that displays the animal’s name. Next, we create the “Dog” class, which inherits the attributes and methods of the “Animal” class. The constructor of the “Dog” class adds a new attribute, “raza”, and calls the constructor of the “Animal” class. We created the “dog1” object as an instance of the “Dog” class and the “talk” method is being called. The “speak” method displays the name of the animal, which in this case is “Fido”. The output of this example will be “The animal says: Fido”. Look:
class Animal:
def __init__(self, name, age):
self.name = name
self.age = age
def speak(self):
print("The animal says:")
print(self.name)
class dog(Animal):
def __init__(self, name, age, raza):
super().__init__(name, age)
self.raza = raza
dog1 = dog("Fido", 3, "Labrador")
dog.falar()
# Output:
# The animal says:
# Fido
These are just a few examples of how the init method can be used to initialize objects in Python. In this sense, the method is a fundamental part of object-oriented programming and can be used in many classes to define the attributes and methods of an object.
How the __init__ method can be used to implement inheritance in Python
The method __init__
can is being used to implement inheritance in Python, which is a way of creating classes that inherit behaviors and attributes from other classes. This is done by creating a new class that inherits from an existing class by using the keyword class
followed by the name of the new class and the name of the base class in quotes.
You can then define the attributes and methods of the new class within the base class using the method to initialize the attributes of the new class. Also, you can override base class methods by using the keyword def
to provide new implementations for those methods in the new class.
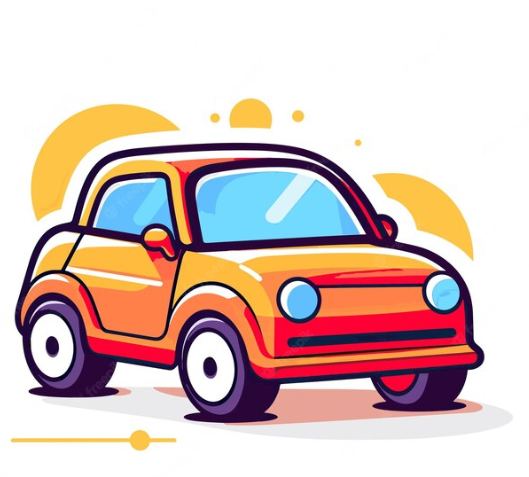
For example, suppose you want to create an automobile class that inherits from a vehicle class. So we can do it like this:
class Car(Vehicle):
def __init__(self, make, model, year):
Vehicle.__init__(self, make, model, year)
self.number_of_doors = 4
self.color = "red"
def start(self):
print("Starting the engine...")
Here, the class Car
inherits from the class Vehicle
and defines a new attribute number_of_doors
and a new method start()
that overrides the method start()
of the class Vehicle
.
Thus, we are using the new class Car
to create car objects that inherit behaviors and attributes from the class Vehicle
and add new behaviors and attributes specific to cars.
Methods similar to method __init__
in Python
There are some methods similar to the method __init__
in Python that are applied to initialize objects:
setattr()
: This method can be applied to define object attributes dynamically, that is, after object creation. Thus, using the method to add custom attributes to an object without having to define an initialization method for the object. Example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
person1 = Person("John Doe", 30)
person1.setattr("age", 40)
print(person1.age) # 40
**kwargs
: We are using this method to pass additional parameters to an initialization method. And so, when we create an object we can be using the method to set additional values for object attributes. It is optional and can be omitted if you don’t need to pass additional parameters. Example:
class Person:
def __init__(self, name, age, **kwargs):
self.name = name
self.age = age
self.kwargs = kwargs
person1 = Person("John Doe", 30, height=180)
print(person1.height) # 180
Tips for writing efficient code with the init method in Python
The method __init__
is a special method in Python that is called automatically when creating a new instance of a class. We use this method to initialize the instance and it can be used to perform any action before the instance is applied. Here are some tips for writing efficient code with the init method in Python:
- Use a body argument to receive instance parameters. This is therefore useful for initializing attributes with values that vary across different instances of the class.
- Use the keyword
self
to reference class attributes and methods. As such, this is needed wherever it is being usedself
, asself
it is a variable automatically passed to the method__init__
. - Initialize all attributes of the class in the method
init
instead of using the keyworddefault
. This makes the code more readable and helps to avoid initialization errors. - Use a block
try-except
to handle initialization errors. Thus, this helps ensure that your class is more robust and resilient. - Use a list to initialize various class attributes. This is useful when you need to initialize several class attributes with values that are similar.
Trends and news regarding the init method in recent versions of Python.
The init method is one of the main features of objects in Python, and recently, there have been some trends and new developments regarding it in recent versions of the language. In that sense, here are some of the main changes and trends:
- Self-registered initialization method: In Python 3.8, added a new self-registered initialization method, which allows developers to define a method
__init__
for objects that will be called automatically when we create an object. Thus, this allows developers to write less code and make objects easier to use. - Custom initialization method: In Python 3.7, added support for custom initialization methods, which allows developers to define a
__init__
custom method for their objects. Thus, this method can include additional code for object initialization, in addition to the standard code. - Use of decorators: Decorators are a way to modify the behavior of an object or method, without changing the source code. So, in Python 3.8, added support for initialization decorators, which allow developers to add Custom behaviors to their objects during initialization.
- Using generic classes: In Python 3.8, added support for generic classes, which allow developers to create classes that can be used with different data types.
- Static initialization method: In Python 3.9, added support for static initialization methods. This way allowing developers to define a
__init__
static method for their objects.