Bash commenting: 3 ways to use comments
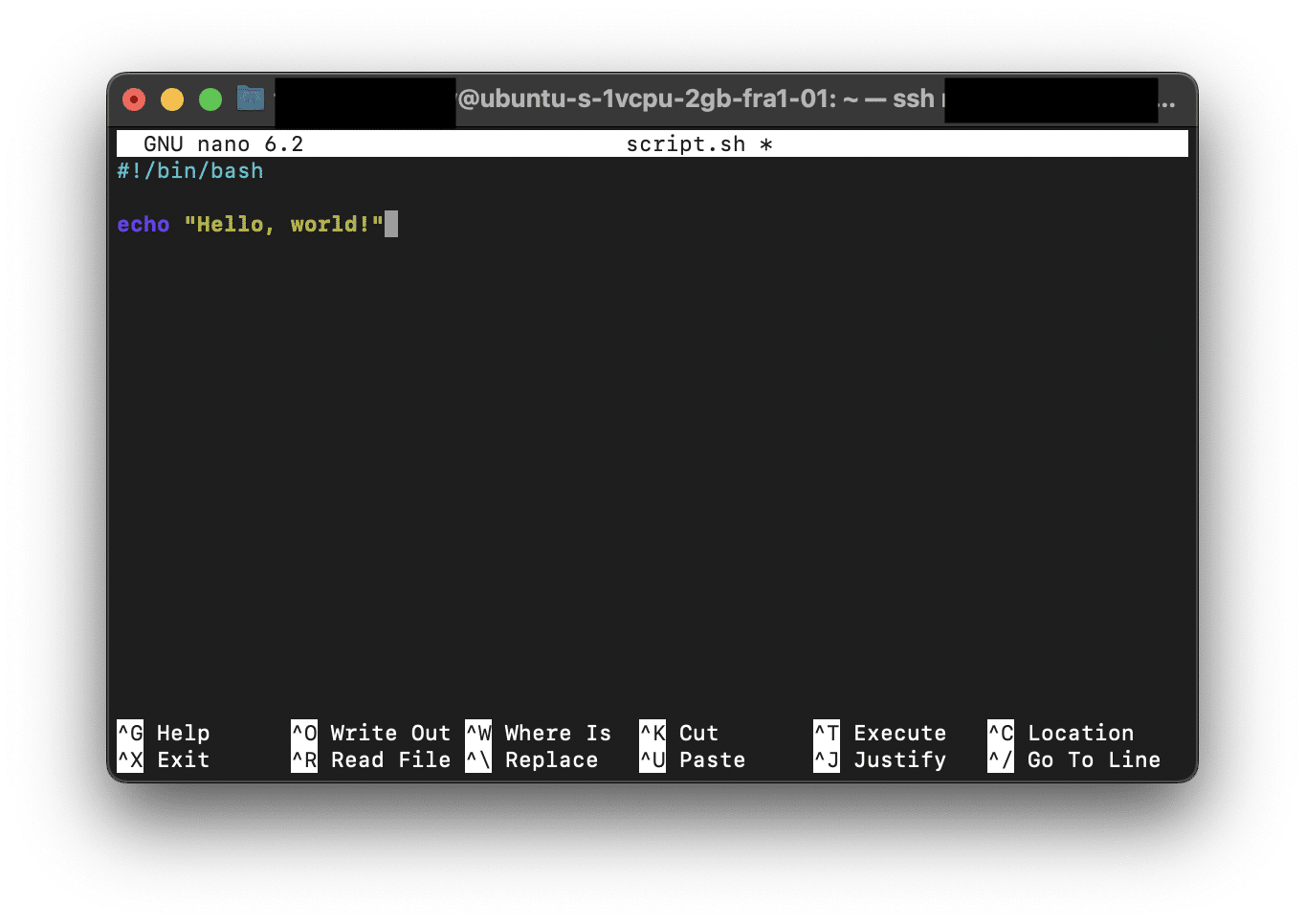
Bash commenting is an important aspect while developing software, as comments provide information and explanations about scripts. These explanations and information not only enable the coder to easily understand their own commands in the future, but also inform other developers about the code. So, in this article, we will explore the use of comments in bash scripts by covering different styles of comments and best practices. In other words, a bash comment won’t be executed. It’s only meant for documenting.
Table of Contents
Bash Commenting Styles
There are two primary styles of comments: single-line comments and multi-line comments
Single-Line bash commenting
Single-line comments are used to add comments to a particular line of code. In Bash, the “#” character denotes the beginning of a single-line comment. Anything after the “#” character on the same line is considered a comment and is not executed by the shell.
# This is a single-line comment.
echo "Hello, World!" # This is another comment.
Here is how the code above looks like in a bash file opened with the Nano command line editor:
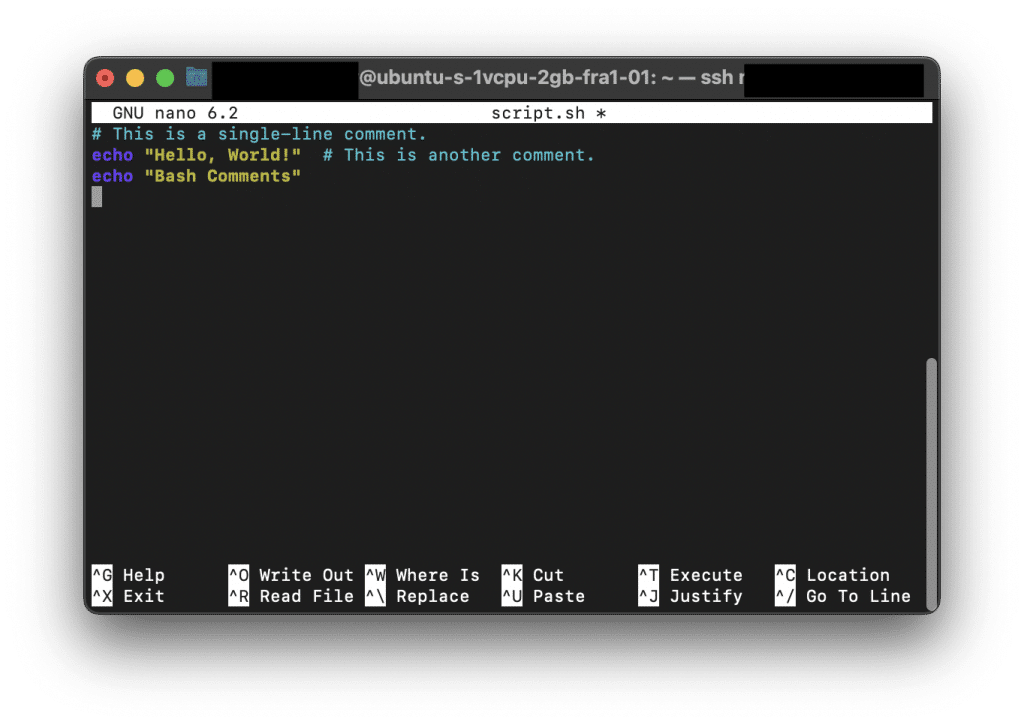
Multi-Line Comments
Bash does not have built-in support for multi-line comments like some other programming languages. However, you can simulate multi-line comments by using multiple single-line comments.
# This is a multi-line comment
# and provides detailed information
# about the script or code block.
echo "Hello, world!"
# This is another comment
# explaining a specific part
# of the code.
Here is how it looks in the nano editor:
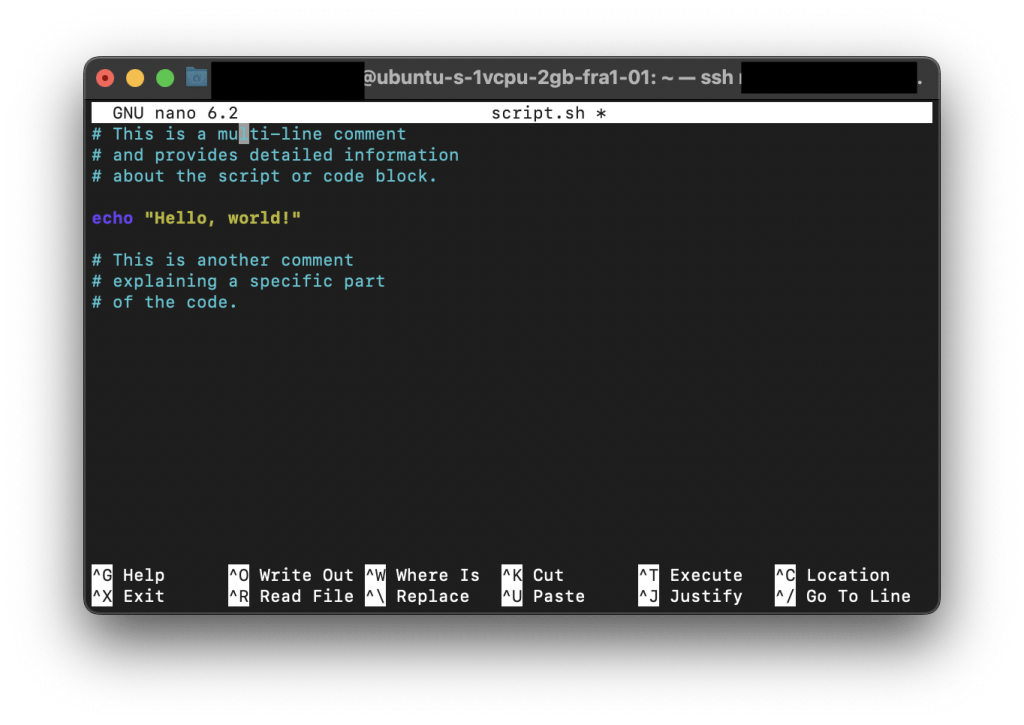
The other way is to use the null command (:
) along with the heredoc notation. Heredoc is often used when you need to pass a block of text or code as an input to a command. In addition to this, it can also be used to create multi-line comments.
: <<COMMENT
This is a multiline block comment.
You can write multiple lines here.
COMMENT
If you paste the above code into an editor, it would look like the image shown below:
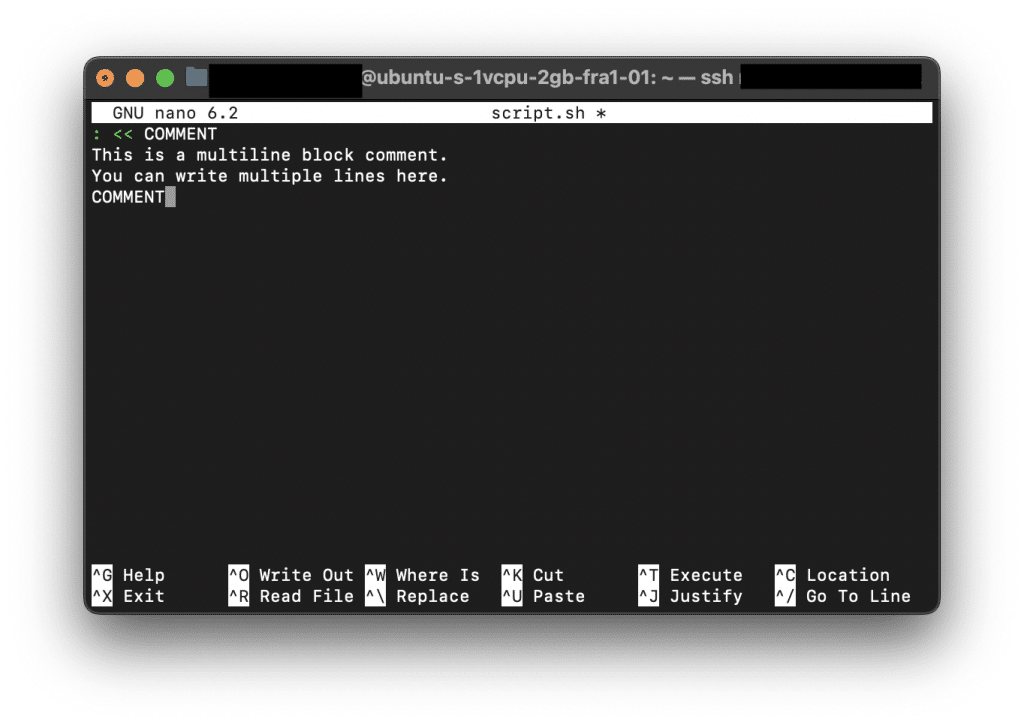
Note that this is a trick to create Multi-line comments, so it is recommended to use Single-Line Comments to create multi-line comments.
An Exception: Shebang
In Bash scripting, there is a special syntax known as shebang or hashbang. It is very similar to a single-line comment as it is denoted by the combination of a hash symbol (#) followed by an exclamation mark (!). However, it is not a comment and is used to inform the system about the interpreter to be used for executing the script.
#!/bin/bash
echo "Hello, world!"
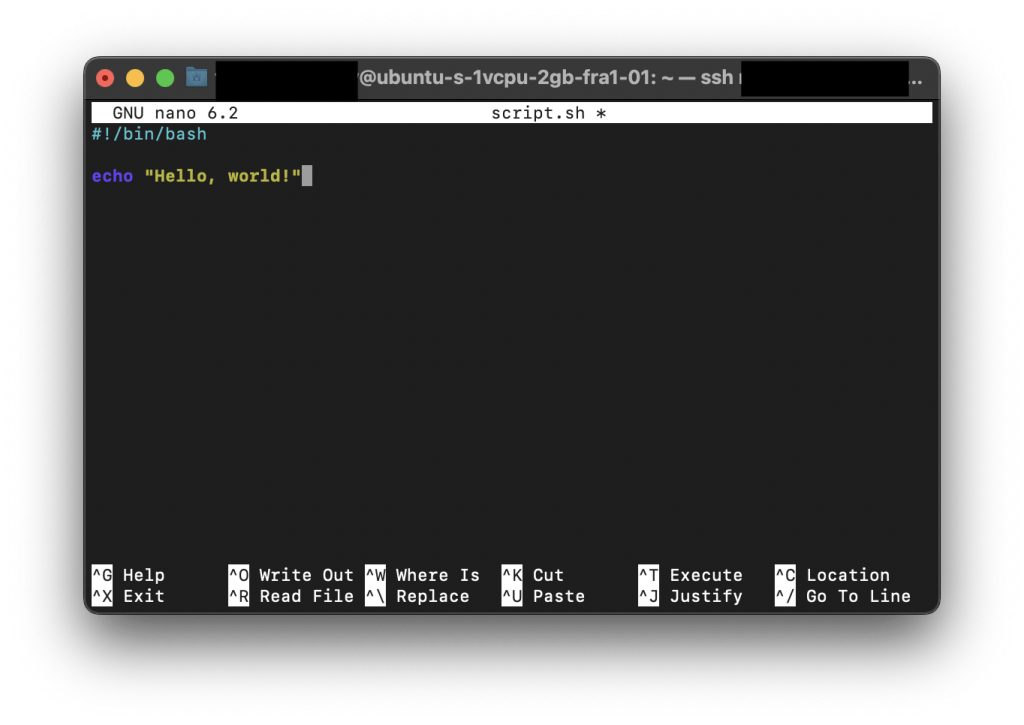
Best Practises For Bash Commenting
Writing comments in bash scripts is essential. At this point, there are some guidelines to consider when adding comments to your Bash scripts:
- Be concise and clear: Write comments that are short but clearly convey the intended message. Avoid unnecessary detail.
- Include a header comment: Begin your script with a header comment. It can provide an overview of the script’s purpose, author, date and more
- Avoid unnecessary comments: Do not specify the obvious in the comments. Focus on explaining the logic behind the code
- Avoid excessive commenting: While comments are helpful, over-commenting can mess up the code and make it harder to read.
- Use comments for documentation: Comments can also serve as a form of documentation providing an overview of the script’s purpose, input/output, dependencies and usage instructions.
FAQ
What is commenting meant for, in programming?
A comment in programming is a piece of text that is added to the source code of a program to provide explanations, clarifications, or documentation about the code. Comments are not executed by the computer and are ignored during the program’s execution. They serve as a way for programmers to communicate with other developers, including themselves, to provide context, describe the purpose of certain code sections, or make notes about the code’s functionality.
Comments are valuable in understanding and maintaining code because they enhance its readability and provide insights into the logic or reasoning behind certain decisions. They can also serve as reminders for future modifications or improvements. Additionally, comments can be used to disable or “comment out” sections of code temporarily, preventing them from being executed without actually removing the code.
In summary, comments in programming are essential for code documentation, collaboration, and code maintenance purposes. They help programmers understand the code’s functionality, facilitate teamwork, and make the code more maintainable and readable in the long run.
Is bash commenting the same as shell script comments?
Yes, in the context of Bash scripting, commenting is the same as in other shell scripting languages. Bash is a popular Unix shell and command language, and it uses the same syntax for comments as other shell scripting languages like sh or csh.
In Bash, you can use the ‘#’ symbol to indicate the start of a comment. Anything written after the ‘#’ symbol on the same line is considered a comment and is ignored by the interpreter when executing the script. Strings, numbers, anything after the # will be ignored by the bash interpreter.
Comments in Bash are used to provide explanations, document the code, or temporarily disable certain lines of code without affecting the script’s functionality.
It’s worth noting that Bash also supports multiline comments using a combination of the ‘:’ (colon) symbol and the ‘<<‘ operator. This syntax allows you to enclose multiple lines of comments within a block, which can be useful for longer explanations or documentation.
In summary, whether you are writing a Bash script or any other shell script, the concept and usage of comments remain the same. They provide a way to add explanatory text or disable code sections without affecting the script’s execution.
How to uncomment a line in bash?
To uncomment a line in a Bash script, you simply need to remove the ‘#’ symbol at the beginning of the line. Here’s how you can do it:
- Open the Bash script file in a text editor of your choice.
- Locate the line that is commented out with the ‘#’ symbol.
- Remove the ‘#’ symbol from the line.
- Save the file.
By removing the ‘#’ symbol, you effectively uncomment the line, allowing it to be interpreted and executed by the Bash interpreter.
It’s important to note that uncommenting a line means that the line of code will be active and executed when the script runs. Therefore, ensure that you uncomment the line only if you intend for it to be part of the script’s functionality.
Conclusion
To sum up, Bash commenting is essential when creating scripts. In fact, although bash only supports single-line comments, multi-line comments can be created with a few tricks. This article has covered how to write comments in bash and best practices for commenting.
Thank you for reading