Compare Strings In Bash
When working with strings in Bash, it is often necessary to compare them in order to execute commands based on their values. In Bash, as in other programming languages, string comparisons are done with the if conditionals and comparison operators. In this article, we’ll cover how these operators are used to compare strings, giving examples of each.
Table of Contents
Comparison Operators
The comparison operators are used to compare the values of strings. They return a boolean value of true or false depending on the comparison result. Here are the most used string comparison operators in Bash.
- Equal (
==
) or (=
): This operator tests if two strings are equal. - Not Equal (
!=
): It checks if two strings are not equal. - Greater Than (
>
): This operator compares two strings alphabetically and checks if the first string is greater than the second. - Less Than (
<
): It compares two strings alphabetically and checks if the first string is less than the second. - Greater Than or Equal To (
>=
): This operator checks if the first string is greater than or equal to the second. - Less Than or Equal To (
<=
): It checks if the first string is less than or equal to the second. - Pattern matching (
=~
): This operator checks if a string matches a specific pattern using regular expressions. - Substring containment(
*
): It checks if a string contains a specific substring, - Empty string (
-z
): This operator checks if a string is empty. - Non-empty string (
-n
): This operator checks if a string is non-empty.
Examples
Now, you can create a bash file with the .sh
extension and open it with a command line editor to compare strings. Here is an example of opening a file with the nano editor available on Linux and MacOs operating systems.
touch comparison.sh // Create a bash file
nano comparison.sh // Open the file
bash comparison.sh // Run the commands in the file
Check If Two Strings Are Equal
To check if two strings are equal, you can use the =
operator with the single brackets []
or the ==
operator with the double square brackets [[ ]]
. Here’s an example:
#!/bin/bash
string1="Hello"
string2="Hello"
if [ "$string1" = "$string2" ]; then
echo "The strings are equal."
fi
if [[ "$string1" == "$string2" ]]; then
echo "The strings are also equal."
fi
When it is executed, the result would look like:
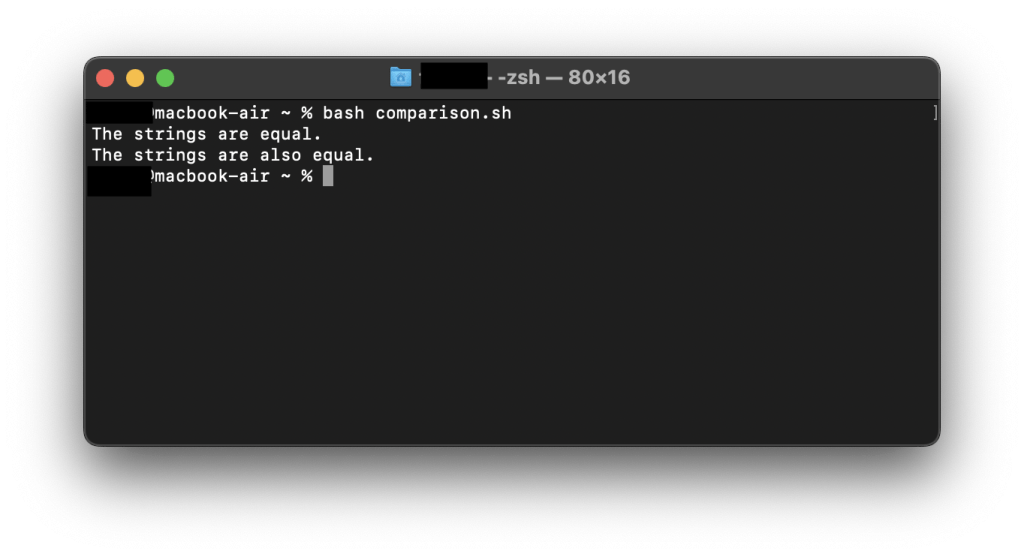
Check If Two Strings Are Not Equal
To check if two strings are not equal, you can use the !=
operator with the single brackets []
or the double square brackets [[ ]]
. Here’s an example:
#!/bin/bash
string1="Hello"
string2="World"
if [ "$string1" != "$string2" ]; then
echo "The strings are not equal."
fi
if [[ "$string1" != "$string2" ]]; then
echo "The strings are also not equal."
fi
In this case, both if conditions will be satisfied, as string1 and string2 are not equal. The output will be:
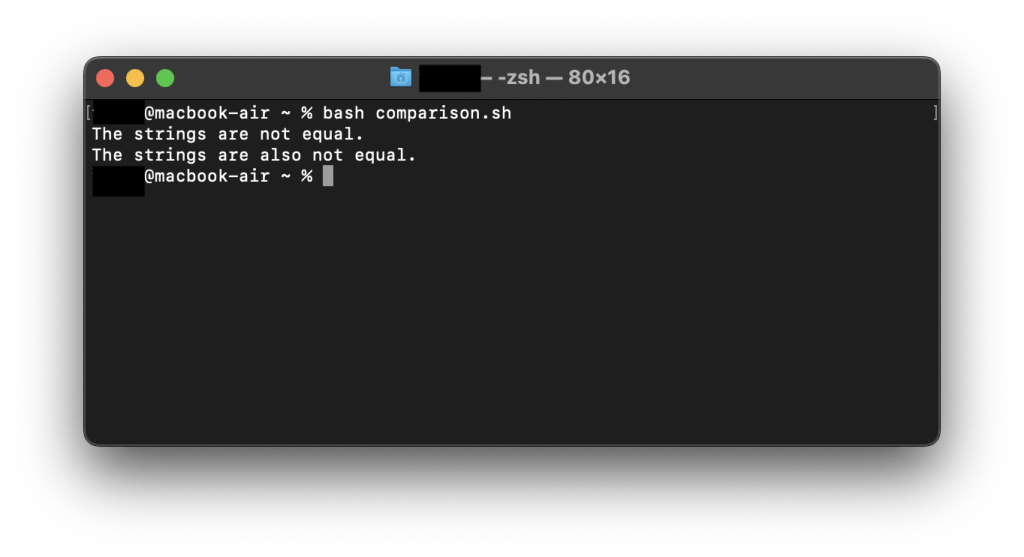
Alphabetical Comparison
To compare strings based on their alphabetical order, you can use the <
, >
, <=
, and >=
operators. Here’s an example:
#!/bin/bash
string1="Apple"
string2="Banana"
if [[ "$string1" < "$string2" ]]; then
echo "string1 comes before string2."
fi
if [[ "$string1" > "$string2" ]]; then
echo "string1 comes after string2."
fi
In this example, the first if
condition will be satisfied since “Apple” comes before “Banana” in alphabetical order. Here is the result:
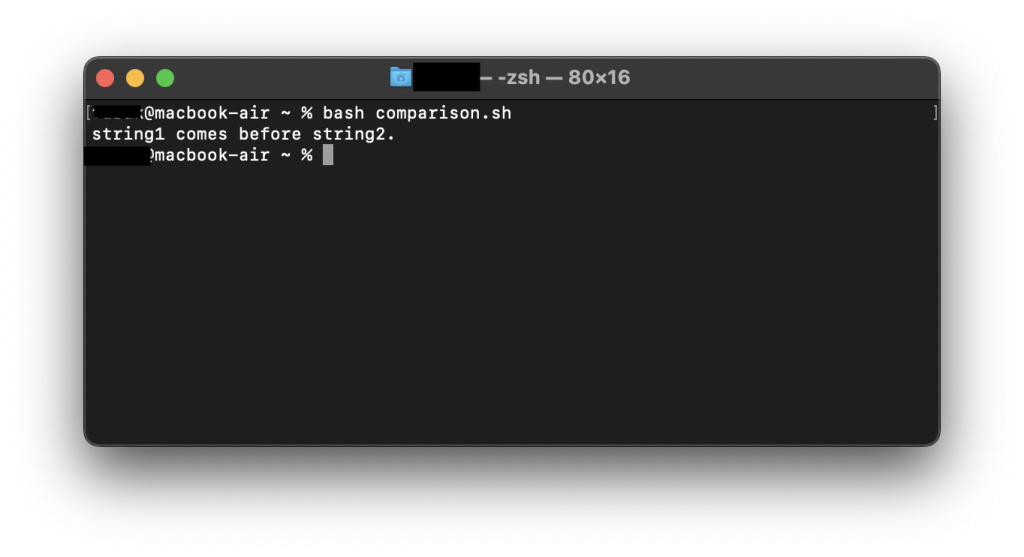
Pattern Matching
The pattern matching operator checks if a string matches a specific pattern using regular expressions. Here is an example of how to check if a string contains only numbers.
#!/bin/bash
string1="234"
if [[ $string1 =~ ^[0-9]+$ ]]; then
echo "String consists of digits only"
else
echo "String contains non-digit characters"
fi
As the string only contains of numbers, the result will be like:
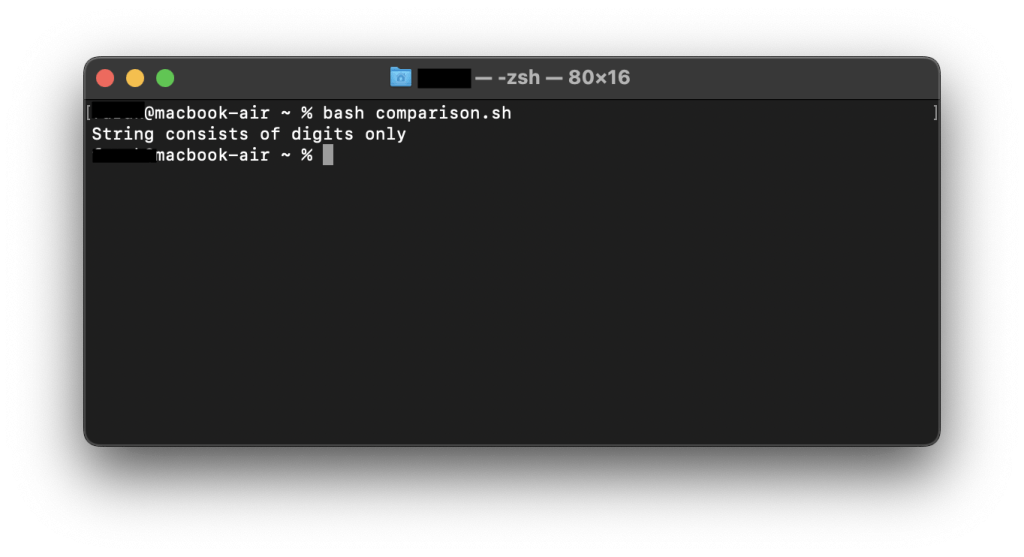
Check If A String Contains A Specific Substring
To check if a string contains a specific substring at the beginning or the end, you can use the *
(asterisk) symbol.
#!/bin/bash
string="HelloWorld"
if [[ "$string" == *ello* ]]; then
echo "String contains 'ello' substring"
fi
if [[ "$string" == Hello* ]]; then
echo "String contains 'Hello' at the beginning"
fi
if [[ "$string" == *World ]]; then
echo "String contains 'World' at the end"
fi
The all conditions will be satisfied and the script will generate the following output:

Check If A String is Empty Or Not
To check if a string is empty or not, you can use the -z
and n
operators. Here is an example:
#!/bin/bash
string=""
if [[ -z "$string" ]]; then
echo "String is empty"
fi
if [[ -n "$string" ]]; then
echo "String is not empty"
fi
Since the string is empty, the output will be:
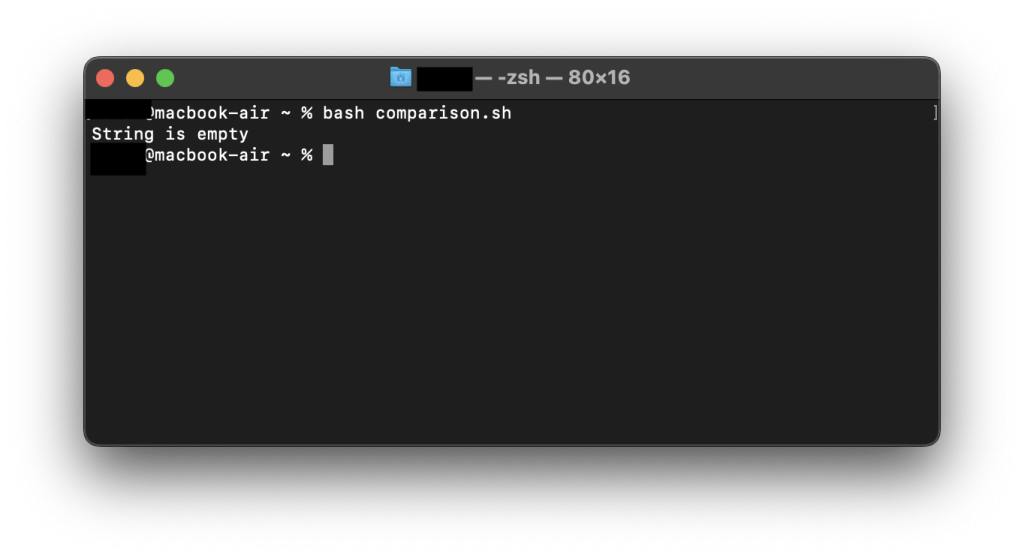
Conclusion
Comparing strings is a common operation when writing Bash scripts. In Bash, there are several operators that we can use for this purpose, as a comment. These operators offer a wide variety of options and they can be combined with conditional statements (if
, elif
, else
) to perform different actions based on the comparison results.
Thank you for reading