Set python: Optimizing data handling
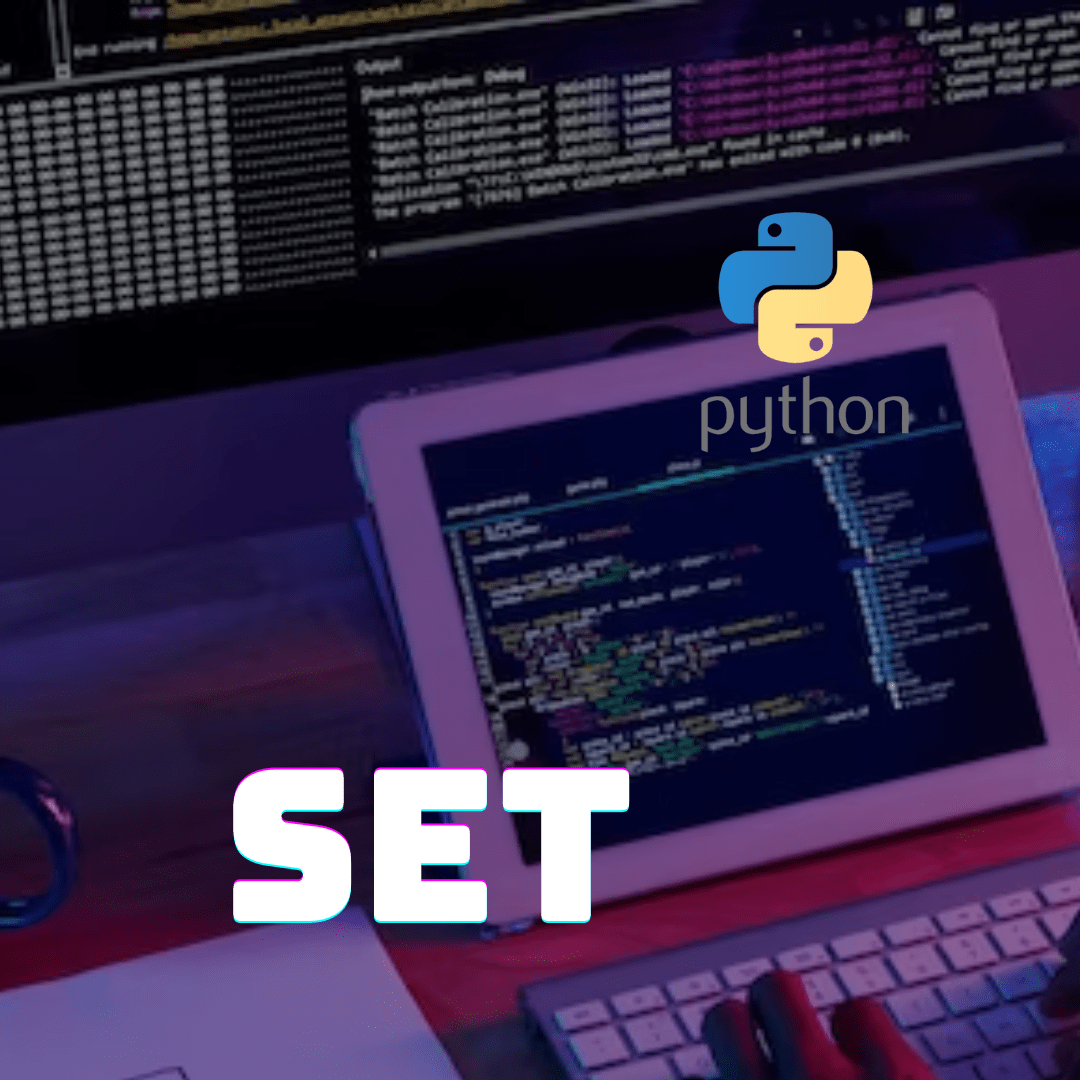
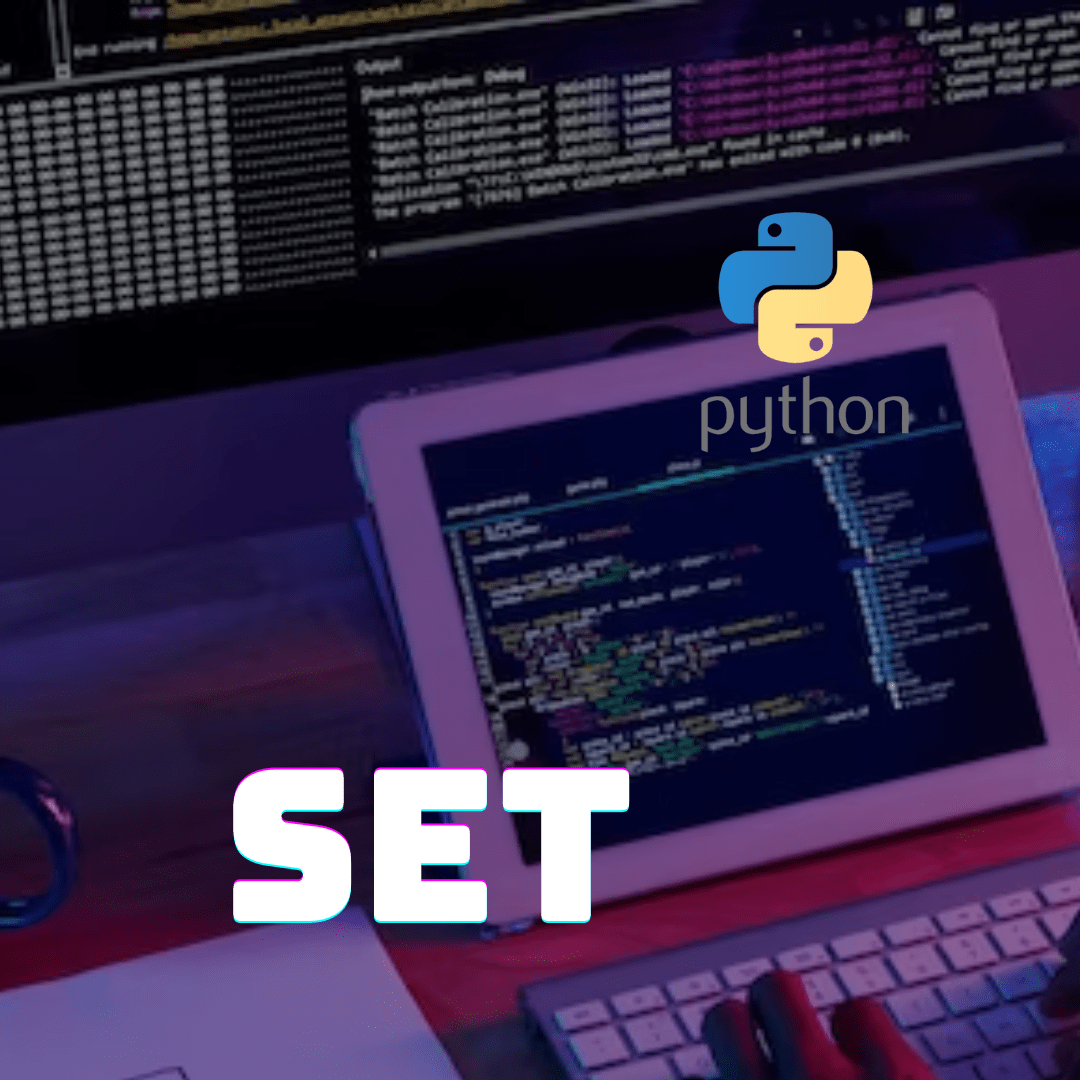
The set in Python is a very useful data structure for handling unique and unordered sets of values. This means that elements cannot be duplicated and the order is not important. Therefore, set are useful for ensuring data integrity, avoiding data replication conflicts, and optimizing performance for some operations.
Set in Python can be created using the function set() when working with lists or tuples . They can be manipulated through methods like union, intersection, difference, empty sets, and others. In addition, Python also supports set comprehensions, which allow you to create sets more concisely and easily.
Sets in Python have a very efficient data structure, as they do not have duplicate elements and allow quick search, insertion and deletion operations. As such, this makes sets an ideal choice for many situations where speed and efficiency are important.
In this article, we’re going to explore sets in Python. Thus, learning about sets, including what they are, how they are represented, and how they can be used to maintain data integrity. Next, we’ll discuss the difference between sets and lists and how they can be manipulated in Python.
Table of Contents
set() function syntax
In Python, the set syntax is based on a set of values with no order or repetitions. You can create a set using square brackets, and add elements using the add()
. Remove elements using the method remove()
, and check if an element is present using the method in
. Also, you can get the cardinality (number of elements) of a set using the len()
.
Examples:
- Creating an empty set:
my_set = set()
print(my_set)
# Output: set()
- Creating a set with multiple elements:
my_set = set([1, 2, 3, 4, 5])
print(my_set)
# Output: {1, 2, 3, 4, 5}
- Adding an element to a set:
my_set = set([1, 2, 3, 4, 5])
my_set.add(6)
print(my_set)
# Output: {1, 2, 3, 4, 5, 6}
- Removing an element from a set:
my_set = set([1, 2, 3, 4, 5])
my_set.remove(3)
print(my_set)
# Output: {1, 2, 4, 5}
- Checking if an element is present in a set:
my_set = set([1, 2, 3, 4, 5])
print(3 in my_set)
# Output: False
print(5 in my_set)
# Output: True
- Get the cardinality of a set:
my_set = set([1, 2, 3, 4, 5])
print(len(my_set))
# Output: 5
Methods and operators used with set in python
In Python, sets are a way of storing collections of unique values. They are useful for performing operations such as unions, intersections, and differences, as well as allowing for membership checking and element removal.
Here are some methods and operators that can be used with sets in Python:
Methods:
add()
: adds an element to the set.
my_set = {1, 2, 3}
my_set.add(4)
print(my_set) # Output: {1, 2, 3, 4}
remove()
: removes an element from the set.
my_set = {1, 2, 3}
my_set.remove(3)
print(my_set) # Output: {1, 2}
discard()
: removes and returns an element from the set.
my_set = {1, 2, 3}
my_set.discard(2)
print(my_set) # Output: {1, 3}
clear()
: removes all elements from the set.
my_set = {1, 2, 3}
my_set.clear()
print(my_set) # Output: set()
pop()
: removes and returns the last element added to the set (if any).
my_set = {1, 2, 3}
my_set.add(4)
my_set.pop()
print(my_set) # Output: {1, 3}
update()
: updates the set with elements from another set.
my_set = {1, 2, 3}
my_set.update({4, 5, 6})
print(my_set) # Output: {1, 2, 3, 4, 5, 6}
union()
: Returns a new set containing all elements from both sets.
set1 = {1, 2, 3}
set2 = {4, 5, 6}
union_set = set1.union(set2)
print(union_set) # Output: {1, 2, 3, 4, 5, 6}
intersection()
: returns a new set containing all elements common to both sets.
set1 = {1, 2, 3}
set2 = {4, 5, 6}
intersection_set = set1.intersection(set2)
print(intersection_set) # Output: {4, 5}
difference()
: Returns a new set containing all the elements of one set but not the other.
set1 = {1, 2, 3}
set2 = {4, 5, 6}
difference_set = set1.difference(set2)
print(difference_set) # Output: {1, 2}
isdisjoint()
: returnsTrue
if the sets have no elements in common.
set1 = {1, 2, 3}
set2 = {4, 5, 6}
if set1.isdisjoint(set2):
print("Sets do not have any elements in common.")
else:
print("Sets have elements in common.")
Operators
+
( union ): Returns a new set containing all elements from both sets.&
( intersection ): Returns a new set containing all elements common to both sets.|
( difference ): Returns a new set containing all the elements of one set but not the other.-
( removing common elements): Returns a new set containing all the elements of one set, except those present in the other set.^
( exclude common elements): Returns a new set containing all elements from both sets, except those present in both.in
( membership check ).
Difference between sets and lists
In Python, sets and lists are different data structures that have different characteristics and purposes. In this topic we will learn how sets are different from lists in python, in terms of structure, data handling and performance.
Structure:
Sets and lists are distinct data structures in Python. A set is a collection of uniques elements, while a list is an ordered collection of elements.
Example:
my_list = [1, 2, 3, 4, 5]
my_set = {1, 2, 3, 4, 5}
In other words, a set does not allow duplicate elements, while a list can contain duplicate elements.
Data manipulation:
Sets are best suited for union, intersection, and difference operations, as these operations are more effective on sets than on lists. Furthermore, sets are more efficient in terms of memory space, as they do not store duplicate elements, while lists can store many duplicate elements.
Example:
# Adding an element at position 2 in the list
my_list.append(6)
# Removing an element at position 3 in the list
my_list.pop(3)
# Adding an element to the set
my_set.add(6)
# Removing an element from the set
my_set.remove(3)
In terms of data manipulation, sets are best suited for membership check operations, element removal, and equality testing. On the other hand, lists are better suited for operations such as accessing elements by index, adding and removing elements, and creating sublists.
Performance:
In terms of performance, sets are faster than lists at operations like union, intersection, and difference. This is because these operations involve comparing and manipulating element references rather than copying data. Furthermore, sets are more efficient in terms of memory space, as they do not store duplicate elements, while lists can store many duplicate elements.
Example:
# Intersection between two lists
list1 = [1, 2, 3, 4, 5]
list2 = [3, 4, 5, 6, 7]
result = list1.intersection(list2)
print(result)
# Output: [3, 4, 5]
# Intersection between two sets
set1 = {1, 2, 3, 4, 5}
set2 = {3, 4, 5, 6, 7}
result = set1.intersection(set2)
print(result)
# Output: {3, 4, 5}
In summary, sets and lists are different data structures in Python, with different characteristics and purposes. Sets are best suited for union, intersection, and difference operations, while lists are best suited for operations such as accessing elements by index, adding and removing elements, and creating sublists. In terms of performance, sets are faster than lists at operations like union, intersection, and difference, and they are more efficient in terms of memory space.
How to use dictionaries and Set in python to optimize data handling
Using sets and dictionaries together can streamline data manipulation in Python, as each data structure offers useful features for different tasks. Sets are useful for storing collections of unique, unordered elements, while dictionaries are useful for storing key-value pairs, where each key is unique.
A common example of using sets and dictionaries together is implementing lookup functions on lists. Instead of creating a list of unique elements, which can be expensive in terms of memory, we can use a set to store the unique elements and a dictionary to store additional information about each element, such as their positions in the original list.
Example:
For example, we can implement a search function on a list of integers using sets and dictionaries as follows:
def search_in_list(lst, target):
unique_elements = set(lst)
index_dict = {}
for element in unique_elements:
if element == target:
return index_dict
index_dict[element] = len(index_dict)
return None
In this example, the function search_in_list()
receives a list of integers and an integer target
to search for. So we first create a set unique_elements
from the list lst
, storing only the unique and unordered elements. Next, we create a dictionary index_dict
, where each key is an element in the list and the value is the index of the element in the original list.
Each element in the list is searched for in the set unique_elements
and, if found, its index is returned. If the element target
is not found in the list, the function returns None
.
In the example, sets are used to store single elements and dictionaries are used to store additional information about each element, such as their positions in the original list. In this way, we can implement a search function in a list efficiently and without spending much in terms of memory.
Furthermore, sets can also be used to implement sorting algorithms such as QuickSort , where elements are divided into sorted sets and then combined to create an ascending or descending order.
In short, the combination of sets and dictionaries can help streamline data manipulation in Python, providing an efficient and flexible way to store and manipulate collections of data.
Using set in data structures in python
In Python, sets are a data structure that allows you to store a set of elements immutably. They are useful when we need to store values that must be unique and we have no need for order.
For data structures, you need to define the operations and methods needed for the specific problem you intend to solve. For example, to use a heap, you need to define the sort method and the fetch method. So, to use a tree, you need to define the search method and the sort method. To use a graph, you must define the search method and the connectivity method. Below we will have each case and examples.
heaps with the set
Heaps are hierarchical data structures that allow you to perform sorting and searching operations faster than other data structures. In Python, we can use heaps to solve sorting and searching problems, such as the largest number in a set of numbers problem.
Here’s an example of how to create a heap in Python using sets. In it, we create a set with the values 1, 2, 3, 4, 5, 6, 7, 8, 9, 10. Then, we create a loop that adds the last element of the set to the set itself, creating a heap. Finally, we print the elements at the top of the heap, which are the highest values. Look:
my_set = set([5, 3, 7, 1, 4, 6, 2, 8, 9, 10])
# create a heap based on the value of the last element
while len(my_set) > 1:
my_set.add(my_set.pop())
# print top of heap
print("The elements at the top of the heap:", sorted(list(my_set)))
trees with the set
Trees are data structures that allow you to perform search and sort operations quickly. In Python, we can use trees to solve searching and sorting problems, such as the largest number in a set of numbers problem.
Here is an example of how to create a tree in Python using set. In the example, we create a set with the values 1, 2, 3, 4, 5, 6, 7. Next, we create the root node with the value 5. Then, we create the child nodes of the root node with the values 2, 3 and 6. Then, we create a child node of the root node with the value 7 and another child node of the root node with the value 8. Finally, we print the tree, which is a data structure represented by a set of elements. Look:
my_set = set([1, 2, 3, 4, 5, 6, 7])
# create the root node
root = 5
# create the child nodes of the root node
my_set.add(2)
my_set.add(3)
my_set.add(6)
# create a child node of the root node
my_set.add(7)
# create another child node of the root node
my_set.add(8)
# create another child node of the root node
my_set.add(9)
# print the tree
print("The tree:", sorted(list(my_set)))
Graphics with the set
Graphs are data structures that allow you to represent relationships between elements. In Python, we can use graphs to solve connectivity and search problems, such as the shortest path problem between two points on a graph.
Here’s an example of how to create a simple graph in Python using sets. In the example, we create a set with the values 1, 2, 3, 4, 5. Next, we create a node with the value 1 and add two child nodes to it. We then create three more child nodes and add a child node to them. Finally, we print the graph, which is a data structure represented by a set of elements. See below:
my_set = set([1, 2, 3, 4, 5])
# create a node
node1 = 1
# add a child node to the node
node2 = 2
my_set.add(node2)
my_set.add(node1)
# create another child node
node3 = 3
my_set.add(node3)
my_set.add(node1)
# create another child node
node4 = 4
my_set.add(node4)
my_set.add(node1)
# create another child node
node5 = 5
my_set.add(node5)
my_set.add(node1)
# print the chart
print("The chart:", sorted(list(my_set)))
Conclusion
In conclusion, Python sets are a useful data structure for storing collections of unique, unordered elements. They can be created using parentheses, the set() method, or the set
. In addition, sets have several methods for manipulating elements, such as addition, removal, intersection, and union. These methods allow sets to be used efficiently and flexibly in a variety of scenarios such as data analysis, algorithm programming, and problem solving. In addition, Python sets are immutable, which guarantees the security of stored data and avoids race condition problems. Because of this, Python sets are a powerful tool for any programmer working with collections of data.