Join SQL: How to use it?
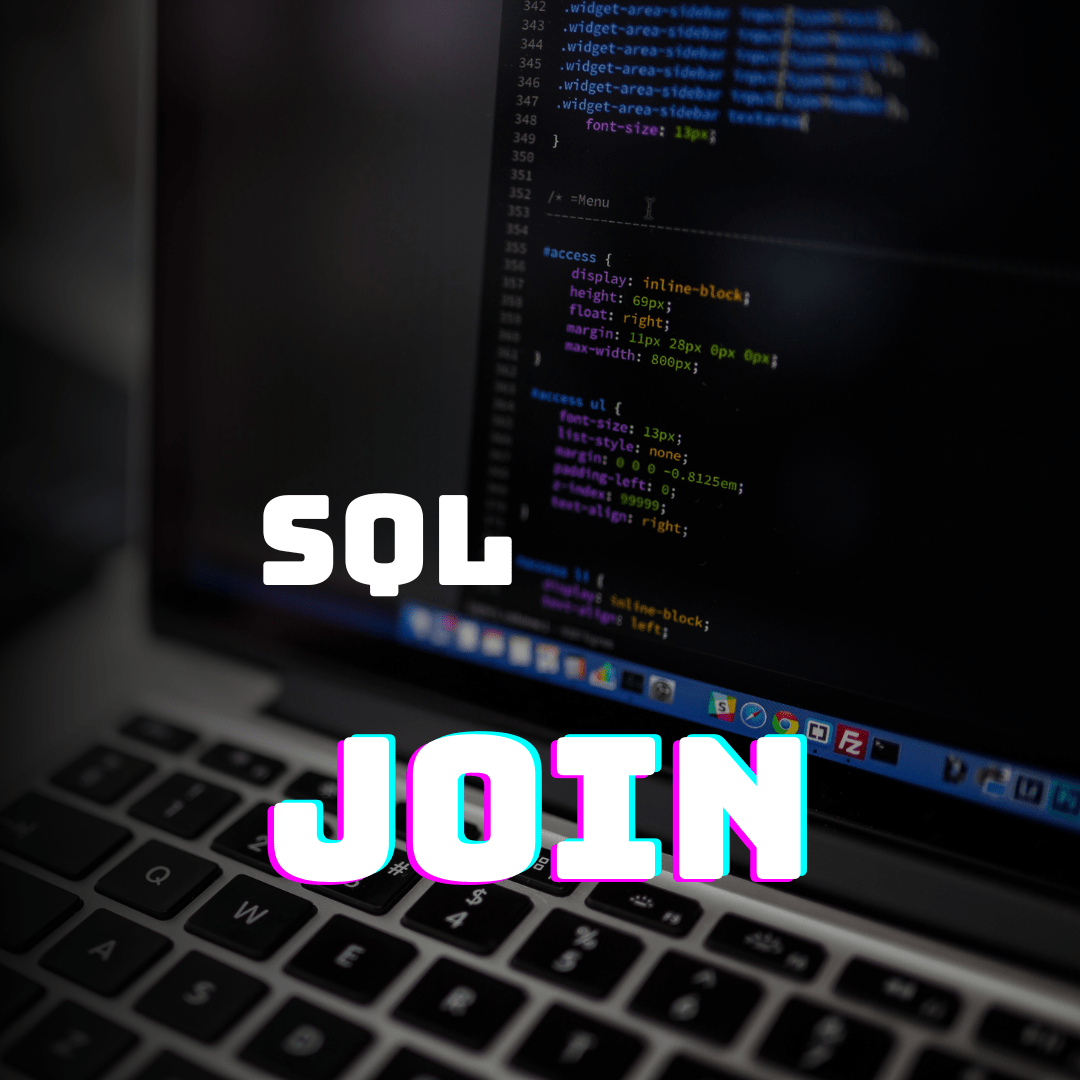
In the world of databases, the “JOIN” command is one of the most powerful tools used in SQL (Structured Query Language) to interact with the data stored in these databases. Therefore, we use JOIN
to combine information from related tables, allowing users to get answers to complex, detailed questions based on multiple columns and rows of data .
In this article, we will cover the JOIN command in SQL, including its different ways of using it, such as INNER JOIN, LEFT JOIN, RIGHT JOIN and FULL JOIN, as well as practical examples and demonstrations of the use of this important command. By understanding and mastering the JOIN
command, you will be able to analyze and explore the data in your database more efficiently and effectively, obtaining accurate and meaningful results for your analyzes and reports.
Table of Contents
What is the JOIN command in SQL?
We use the JOIN command in SQL to combine information from two or more tables into a single query. Thus, allowing us to obtain related information from multiple tables based on a common column.
The JOIN
command is extremely useful in situations where you need to obtain information from different tables to perform more comprehensive analyses. For example, we use JOIN
to combine information from a customer table and an order table to get an overview of which customers purchased which products.
There are several types of JOINs
available, including INNER JOIN
, LEFT JOIN
, RIGHT JOIN
, and FULL OUTER JOIN
. Therefore, each of these types of JOINs
has its own operating rules that we use in different situations.
INNER JOIN is the most common type of JOIN
and we use it to combine only the rows that match in both tables. LEFT JOIN
, on the other hand, combines all the rows in the table on the left with the rows that correspond in the table on the right. The RIGHT JOIN
is similar, but it combines all rows in the right table with the corresponding rows in the left table. The FULL OUTER JOIN combines all rows from both tables, regardless of whether there is a match or not.
Types of JOINs
There are several types of JOINs
available in SQL, each with its own operating rules and specific uses. Here are more details about the types of JOINs:
INNER JOIN
This is the most common type of JOIN
and is used to combine only those rows that match in both tables. For example, the following SQL
command combines the “customers” and “orders” tables based on the “customer_id” column:
SELECT customers.customer_name, orders.order_date
FROM customers
INNER JOIN orders
ON customers.customer_id = orders.customer_id;
LEFT JOIN
LEFT JOIN combines all rows in the left table with the corresponding rows in the right table. If there is no match, the table columns on the right will be filled with default values such as NULL. For example, the following SQL
command combines the “customers” table with the “orders” table, leaving the “orders” table empty records when there is no match:
SELECT customers.customer_name, orders.order_date
FROM customers
LEFT JOIN orders
ON customers.customer_id = orders.customer_id;
RIGHT JOIN
The RIGHT JOIN
is similar to the LEFT JOIN
, but it combines all the rows in the right table with the corresponding rows in the left table. If there is no match, the left table columns will be filled with default values such as NULL. For example, the following SQL
command matches the “customers” table with the “orders” table, leaving the “customers” table empty records when there is no match:
SELECT customers.customer_name, orders.order_date
FROM customers
RIGHT JOIN orders
ON customers.customer_id = orders.customer_id;
FULL OUTER JOIN
The FULL OUTER JOIN
is used to combine all rows from both tables, regardless of whether there is a match or not. It is similar to LEFT JOIN and RIGHT JOIN, but it combines the left and right table rows, respectively. If there is no match, the columns in the table that do not match will be filled with default values such as NULL. For example, the following SQL command combines the “customers” table with the “orders” table in a FULL OUTER JOIN:
SELECT customers.customer_name, orders.order_date
FROM customers
FULL OUTER JOIN orders
ON customers.customer_id = orders.customer_id;
This command will match all rows from the “customers” table with all rows from the “orders” table, regardless of whether there is a match or not. Thus, if there is no match, the columns in the “orders” table will be filled with default values, such as NULL.
Therefore, each type of JOIN has its own operating rules and is used in different situations. It’s important to choose the appropriate JOIN
type for the problem you’re trying to solve and be careful to avoid common mistakes like subquerying and duplicates.
How to use the JOIN command in SQL to combine tables and get related information
The JOIN command in SQL is used to combine rows from two or more tables based on a common column. So, here is an example of how to use the JOIN
command in SQL
to combine two tables and obtain related information:
Suppose you have two tables, “Clients” and “Orders”, with the following columns:
“Clients” table:
- ClientID (primary key)
- Name
- Address
“Orders” table:
- OrderID (primary key)
- ClientID (foreign key)
- OrderDate
- TotalAmount
To combine the “Clients” and “Orders” tables and obtain related information, we use the JOIN INNER JOIN command as follows:
SELECT Clients.Name, Clients.Address, Orders.OrderDate, Orders.TotalAmount
FROM Clients
INNER JOIN Orders
ON Clients.ClientID = Orders.ClientID;
This way, combining the “Clients” and “Orders”. Tables based on the “ClientID” column and will return the “Name”, “Address”, “OrderDate” and “TotalAmount” columns for all rows that have common values in the column “ClientID”.
Therefore, we use other types of JOIN
such as LEFT JOIN, RIGHT JOIN, FULL OUTER JOIN, combining rows from different tables based on different criteria. Therefore, it is important to choose the appropriate JOIN type for your specific situation.
Examples of using the JOIN command in SQL to solve specific problems
The JOIN
command in SQL is a powerful and versatile tool that can be used to solve a wide variety of specific problems. Here are some examples of how the JOIN command in SQL can be used to solve different problems:
- Sales analysis: One of the main applications of JOIN in SQL is sales analysis. Thus, we use
JOIN
by combining information from a product table with a sales table, obtaining an overview of which products are being sold and in what quantity. For example:
SELECT products.product_name, sales.sale_date
FROM products
INNER JOIN sales
ON products.product_id = sales.product_id;
- Data Mapping: We also use JOIN to map data from one table to another. For example, you can use JOIN to map customer addresses to sales information. For example:
SELECT customers.customer_name, orders.order_date
FROM customers
LEFT JOIN orders
ON customers.customer_id = orders.customer_id;
- Data Filtering: In this case, we use JOIN to filter data as well. For example, you can use
JOIN
to combine information from a table with a filter table to get only the records that match a certain filter. For example:
SELECT orders.order_date
FROM orders
INNER JOIN filters
ON orders.order_date >= filters.start_date AND orders.order_date <= filters.end_date;
- Performance analysis: we also use JOIN for performance analysis. For example, you can use
JOIN
to combine information from a performance table with a users table to get an overview of which users are causing poor performance. For example:
SELECT users.user_name, performance.response_time
FROM users
INNER JOIN performance
ON users.user_id = performance.user_id;
Join in sets with the other commands
Now we will see examples of how to use JOIN commands in conjunction with other commands in SQL
JOIN and UPDATE
Suppose you have two tables, “customers” and “orders”. Where the “customers” table contains information about customers and the “orders” table contains information about orders placed by customers. The “customers” table has the following columns: “customer_id”, “name” and “email”. While the “orders” table has the following columns: “order_id”, “customer_id” and “order_total”.
To update a specific customer’s email, we use the UPDATE command as follows:
UPDATE customers
SET email = 'novo_email@exemplo.com'
WHERE customer_id = 1;
This will update the customer email with ID 1 to “new_email@example.com ” .
To combine the two tables and display information about orders placed by each customer, we use the JOIN
command as follows:
SELECT customers.name, orders.order_total
FROM customers
JOIN orders
ON customers.customer_id = orders.customer_id;
This way, combining the “customers” and “orders” tables based on the “customer_id” column and will display the customer name and order total for each result line.
JOIN and COUNT
Suppose you have two tables, “customers” and “orders”. Where the “customers” table contains information about customers and the “orders” table contains information about orders placed by customers. The “customers” table has the following columns: “customer_id”, “name” and “email”, while the “orders” table has the following columns: “order_id”, “customer_id” and “order_total”.
To count the number of orders placed by each customer, you can use the JOIN
and COUNT command as follows:
SELECT customers.name, COUNT(orders.order_id) as num_orders
FROM customers
JOIN orders
ON customers.customer_id = orders.customer_id
GROUP BY customers.name;
This way, the example will combine the “customers” and “orders” tables based on the “customer_id” column and group the results by customer name. The “num_orders” column will count the number of orders placed by each customer.
So these are just a few examples of how we use the JOIN command in SQL to solve specific problems. The power of JOIN
in SQL is that it allows you to get related information from multiple tables based on a common column, which can help you make more informed decisions.
Good practices for using the JOIN command in SQL
We use the JOIN command in SQL to combine rows from two or more tables based on a common column. Here are some best practices for using the JOIN command in SQL:
1. Choose the appropriate JOIN type
There are several types of JOINs available in SQL, including INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL OUTER JOIN. This way, in each specific situation we have a type of JOIN. For example, we use INNER JOIN
to combine rows that have common values in both tables. While we use LEFT JOIN
to combine all rows in the left table with rows in the right table that have common values.
2. Choose the appropriate JOIN order
The order in which the JOINs
are being performed can affect the result of the query. It is recommended to place narrower JOINs
in the left part of the query and broader JOINs
in the right part.
3. Use indexes appropriately
When using JOINs in complex queries, it is important to use indexes appropriately to optimize query performance. So make sure you create indexes on all the columns that we use in the JOIN
and on all the columns that are being used to restrict the query.
4. Avoid unnecessary JOINs
When possible, avoid using unnecessary JOINs. For example, instead of doing a JOIN
between two tables to get a list of names and addresses, you can first get a list of names and then a list of addresses, and then combine the two lists using a JOIN
.
5. Use subqueries
If you need to make a complex query that involves multiple tables, consider using subqueries. This can help simplify the query and improve performance.
6. Consider optimizing complex queries
When you are dealing with complex queries that involve multiple tables and JOINs
, it is important to consider query optimization to improve performance.
These are some of the best practices for using the JOIN command in SQL efficiently and optimally. It is important to remember that each query is unique and may require different approaches depending on the task specifications.
Points of attention when using the JOIN command in SQL
When using the JOIN
command in SQL, it is important to keep some points in mind to avoid problems with the query. Some of these points are:
- Subquery prevention: When you use a subquery in place of a JOIN, it can be less efficient than a JOIN. In this sense, it should be due to the fact that the subquery must be executed separately before being used in the
JOIN
. To avoid this, use JOIN instead of subqueries. - Elimination of duplicates: When we use a JOIN to combine two tables, we must be careful to avoid duplicating rows. Thus, causing a mystery in the column that is being used to make the JOIN. Make sure the column used to do the JOIN has unique values in both tables.
- Order of JOINs: The order in which we perform JOINs can affect the result of the query. Therefore, it is recommended to place the narrower
JOINs
in the left part of the query and the broader JOINs in the right part. This can help avoid issues with query optimization. - Choosing the appropriate JOIN type: Choosing the appropriate JOIN type is important to avoid problems with the query. For example, if we are combining two tables based on a column of data, use a JOIN INNER. If we are combining two tables based on a foreign key column, use a JOIN FOREIGN KEY.
- Using indexes: Make sure to create indexes on the columns used in the JOIN to optimize the query. This can help avoid issues with query performance.
These are some of the points to pay attention to when using the JOIN command in SQL. By following these tips, we can avoid problems with the query and obtain accurate and efficient results.
Conclusion
The JOIN command in SQL is a very important tool for data analysts and software developers. Therefore, we can combine tables and obtain related information efficiently and accurately. Good practices for using the JOIN command include choosing the appropriate JOIN
type, using indexes appropriately, avoiding subqueries, and optimizing complex queries. Furthermore, it is important to keep some points of attention in mind, such as preventing subqueries and eliminating duplicates.
By following these tips, it is possible to use the JOIN
command in SQL efficiently and obtain accurate and efficient results. Thus, this makes the JOIN
command a valuable tool for analyzing and processing large amounts of data, helping to make strategic decisions and improve a company’s operational efficiency.
In summary, the JOIN
command in SQL is an essential tool for those who work with data and is being mastered by professionals in the field. However, it is important to study other important commands in SQL
, such as UPDATE and COUNT, to keep the data up to date and obtain accurate statistics on the data.